python 视频抽帧
时间: 2023-02-08 08:06:49 浏览: 172
可以使用 Python 库中的 OpenCV 和 ffpyplayer 进行视频抽帧。
1. 使用 OpenCV:
```
import cv2
# Open the video file
video = cv2.VideoCapture("path/to/video.mp4")
# Get the frames per second (fps) of the video
fps = video.get(cv2.CAP_PROP_FPS)
# Get the total number of frames in the video
num_frames = int(video.get(cv2.CAP_PROP_FRAME_COUNT))
# Choose the interval for frames to be extracted (e.g. every 10th frame)
frame_interval = 10
# Initialize a counter for the current frame
current_frame = 0
# Loop through the frames of the video
while True:
# Grab the current frame
ret, frame = video.read()
# Break the loop if the video has ended
if not ret:
break
# Increment the frame counter
current_frame += 1
# If the current frame is an interval frame, save it
if current_frame % frame_interval == 0:
cv2.imwrite("path/to/save/frame{}.jpg".format(current_frame), frame)
# Release the video capture object
video.release()
```
2. 使用 ffpyplayer
```
import ffpyplayer
# Open the video file
video = ffpyplayer.Player("path/to/video.mp4")
# Get the frames per second (fps) of the video
fps = video.get_fps()
# Get the total number of frames in the video
num_frames = video.get_metadata()['duration']*fps
# Choose the interval for frames to be extracted (e.g. every 10th frame)
frame_interval = 10
# Initialize a counter for the current frame
current_frame = 0
# Loop through the frames of the video
while video.get_frame():
# Grab the current frame
video.get_frame()
# Increment the frame counter
current_frame += 1
# If the current frame is an interval frame, save it
if current_frame % frame_interval == 0:
video.save_frame("path/to/save/frame{}.jpg".format(current_frame))
```
以上两个代码示例都是每隔10帧抽取一帧。记得根据你的视频帧数和视频长度来调整抽取间隔。
阅读全文
相关推荐
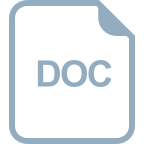
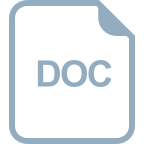
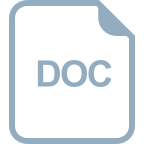







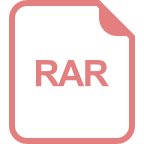
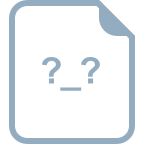





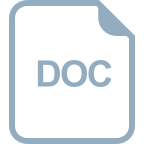
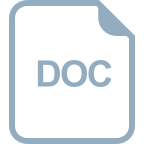