我有一个image to text的图片字幕的程序,即输入图片的路径,然后输出图片描述,以文本的显示,现在我想用web继续部署,使用Django的框架,写出详细的代码、解释、和目录。
时间: 2024-06-11 12:05:05 浏览: 162
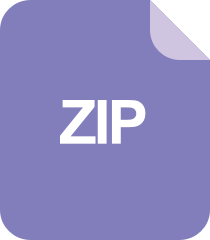
image_to_text
首先,需要在Django项目中创建一个app作为图片字幕的功能模块,可以命名为“caption”。
在“caption” app中,需要创建以下文件和文件夹:
1. models.py:定义数据库表,用于存储图片和对应的文字描述。
2. views.py:实现视图函数,用于处理用户的请求并返回响应。
3. templates文件夹:包含HTML模板文件,用于渲染页面并显示结果。
4. static文件夹:包含静态资源文件,如CSS、JavaScript和图片等。
接下来,需要安装必要的Python库,包括Django、Pillow和pytesseract:
```
pip install django
pip install pillow
pip install pytesseract
```
在models.py中,定义一个模型类,用于存储图片和对应的文字描述:
```python
from django.db import models
class Caption(models.Model):
image = models.ImageField(upload_to='images/')
text = models.TextField()
```
在views.py中,实现一个视图函数,用于处理用户上传图片并返回文字描述:
```python
from django.shortcuts import render
from django.core.files.storage import FileSystemStorage
from django.conf import settings
from PIL import Image
import pytesseract
from .models import Caption
def caption(request):
if request.method == 'POST' and request.FILES['image']:
uploaded_file = request.FILES['image']
fs = FileSystemStorage()
filename = fs.save(uploaded_file.name, uploaded_file)
image_url = fs.url(filename)
image_path = settings.MEDIA_ROOT + image_url
image = Image.open(image_path)
text = pytesseract.image_to_string(image)
caption = Caption(image=uploaded_file, text=text)
caption.save()
return render(request, 'caption.html', {'image_url': image_url, 'text': text})
return render(request, 'caption.html')
```
在templates文件夹中,创建一个HTML模板文件caption.html,用于渲染页面并显示结果:
```html
<!DOCTYPE html>
<html>
<head>
<title>Image Caption</title>
<link rel="stylesheet" type="text/css" href="{% static 'css/style.css' %}">
</head>
<body>
<h1>Image Caption</h1>
<form method="POST" enctype="multipart/form-data">
{% csrf_token %}
<input type="file" name="image">
<button type="submit">Upload</button>
</form>
{% if text %}
<img src="{{ image_url }}">
<p>{{ text }}</p>
{% endif %}
</body>
</html>
```
在static文件夹中,创建一个CSS样式文件style.css,用于美化页面:
```css
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
}
h1 {
text-align: center;
}
form {
display: flex;
flex-direction: column;
align-items: center;
margin-top: 50px;
}
input[type="file"] {
margin-bottom: 20px;
}
button[type="submit"] {
background-color: #4CAF50;
color: white;
border: none;
padding: 10px 20px;
border-radius: 5px;
cursor: pointer;
}
img {
display: block;
margin: 20px auto;
max-width: 100%;
height: auto;
}
p {
text-align: center;
font-size: 24px;
font-weight: bold;
}
```
最后,在Django项目的urls.py中,添加一个URL路由,将用户的请求转发到“caption” app中的视图函数:
```python
from django.urls import path
from caption.views import caption
urlpatterns = [
path('caption/', caption, name='caption'),
]
```
现在,可以在Django项目中启动服务器并访问“http://localhost:8000/caption/”页面,上传一张图片并得到对应的文字描述。
阅读全文
相关推荐
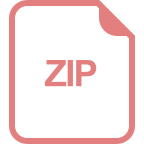
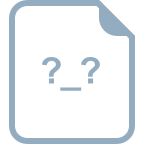
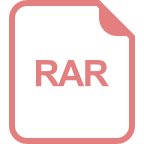
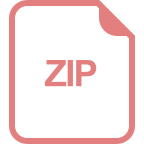
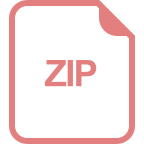
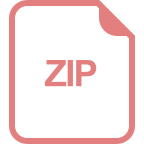
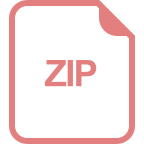
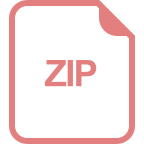
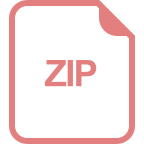
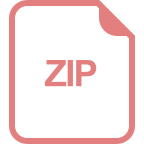
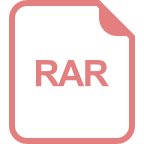
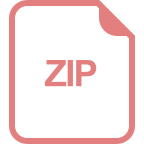
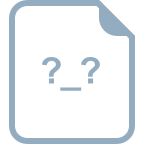
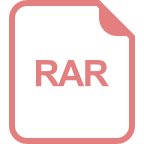
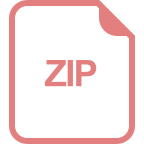
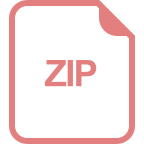