请通过继承的方法创建一个多线程程序,并用 Runnable 接口创建一个多线程程序,最后建立两个线程 a 和 b , a 线程每隔0.1s输出一个' a ',共输出10个' a : b 线程每隔0.2s输出一个 b ,共输出10个。
时间: 2024-01-24 22:17:14 浏览: 29
使用继承方式创建多线程程序:
```java
public class MyThread extends Thread {
private String name;
private int count;
public MyThread(String name, int count) {
this.name = name;
this.count = count;
}
@Override
public void run() {
for (int i = 0; i < count; i++) {
System.out.print(name + " ");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
使用 Runnable 接口创建多线程程序:
```java
public class MyRunnable implements Runnable {
private String name;
private int count;
public MyRunnable(String name, int count) {
this.name = name;
this.count = count;
}
@Override
public void run() {
for (int i = 0; i < count; i++) {
System.out.print(name + " ");
try {
Thread.sleep(200);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
主函数中启动两个线程:
```java
public static void main(String[] args) {
Thread a = new MyThread("a", 10);
Thread b = new Thread(new MyRunnable("b", 10));
a.start();
b.start();
}
```
相关推荐
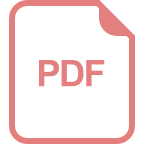
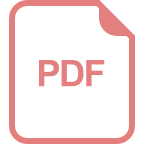
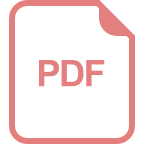














