基于pytorch,帮我编写一个计算置信度的函数代码,其数据为时序数据
时间: 2024-03-22 10:38:55 浏览: 90
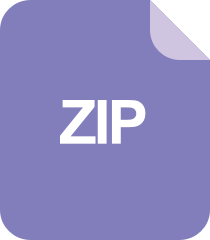
基于pytorch实现的时间序列预测训练框架
好的,以下是一个基于 PyTorch 计算时序数据置信度的代码示例,供您参考:
```python
import torch
import numpy as np
# 定义模型
class LSTMModel(torch.nn.Module):
def __init__(self, input_dim, hidden_dim, num_layers, output_dim):
super(LSTMModel, self).__init__()
self.hidden_dim = hidden_dim
self.num_layers = num_layers
self.lstm = torch.nn.LSTM(input_dim, hidden_dim, num_layers, batch_first=True)
self.fc = torch.nn.Linear(hidden_dim, output_dim)
def forward(self, x):
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_dim).requires_grad_()
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_dim).requires_grad_()
out, (hn, cn) = self.lstm(x, (h0.detach(), c0.detach()))
out = self.fc(out[:, -1, :])
return out
# 计算置信度函数
def confidence_score(model, input_data):
# 将输入数据转换为 PyTorch 张量
input_tensor = torch.Tensor(input_data)
# 计算模型预测结果
with torch.no_grad():
output = model(input_tensor.unsqueeze(0))
prediction = torch.nn.functional.softmax(output, dim=1).numpy()[0] # 将输出转换为概率分布
# 计算置信度
max_prob = np.max(prediction)
confidence = max_prob / np.sum(prediction)
return confidence
# 测试数据
input_data = np.random.randn(10, 3) # 生成10个长度为3的随机向量
# 实例化模型
input_dim = 3
hidden_dim = 4
num_layers = 2
output_dim = 2
model = LSTMModel(input_dim, hidden_dim, num_layers, output_dim)
# 计算置信度
confidence = confidence_score(model, input_data)
print("置信度:", confidence)
```
在这个示例中,我们首先定义了一个 LSTM 模型,用于处理时序数据。然后,我们编写了一个 `confidence_score` 函数,用于计算模型对给定输入数据的置信度。具体实现方式是,首先将输入数据转换为 PyTorch 张量,然后使用模型预测输入数据的类别概率分布,最后计算概率分布中最大值的比例作为置信度。
最后,我们使用随机生成的数据进行测试,并输出计算得到的置信度。这个示例只是一个简单的示例,实际使用中需要根据具体模型和数据进行调整。
阅读全文
相关推荐
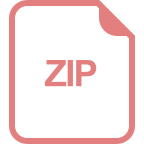
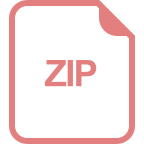

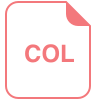
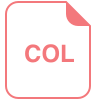
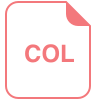
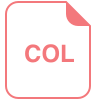
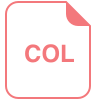
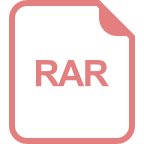
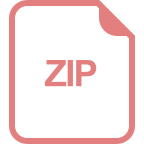
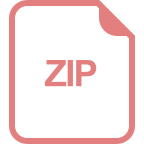
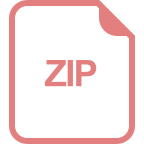
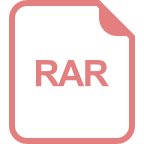
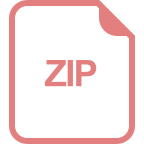
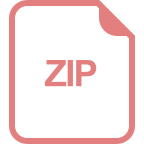
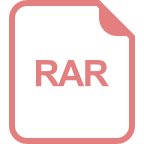