JAVA文件搜索系统连接MysqlGUI界面代码
时间: 2023-11-21 07:05:33 浏览: 37
以下是一个简单的Java文件搜索系统连接MySQL数据库并使用GUI界面的示例代码:
```java
import java.sql.*;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class FileSearch extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
JLabel l1, l2;
JTextField tf1, tf2;
JButton btn1, btn2;
JTable j;
FileSearch() {
setTitle("File Search System");
setSize(700, 700);
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
l1 = new JLabel("Search Path:");
l1.setBounds(50, 50, 100, 30);
tf1 = new JTextField();
tf1.setBounds(150, 50, 250, 30);
l2 = new JLabel("Search Keyword:");
l2.setBounds(50, 100, 100, 30);
tf2 = new JTextField();
tf2.setBounds(150, 100, 250, 30);
btn1 = new JButton("Search");
btn1.setBounds(450, 50, 100, 30);
btn1.addActionListener(this);
btn2 = new JButton("Clear");
btn2.setBounds(450, 100, 100, 30);
btn2.addActionListener(this);
add(l1);
add(tf1);
add(l2);
add(tf2);
add(btn1);
add(btn2);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == btn1) {
String path = tf1.getText();
String keyword = tf2.getText();
try {
Class.forName("com.mysql.jdbc.Driver");
Connection con = DriverManager.getConnection("jdbc:mysql://localhost:3306/files", "root", "password");
Statement stmt = con.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM filesearch WHERE path LIKE '%" + path + "%' AND content LIKE '%" + keyword + "%'");
String[] columns = {"File Name", "Path", "Content"};
String[][] data = new String[100][3];
int i = 0;
while (rs.next()) {
data[i][0] = rs.getString("name");
data[i][1] = rs.getString("path");
data[i][2] = rs.getString("content");
i++;
}
j = new JTable(data, columns);
j.setBounds(50, 200, 600, 400);
JScrollPane sp = new JScrollPane(j);
add(sp);
con.close();
} catch (Exception ex) {
System.out.println(ex);
}
} else if (e.getSource() == btn2) {
tf1.setText("");
tf2.setText("");
}
}
public static void main(String[] args) {
new FileSearch();
}
}
```
在此示例中,我们使用了MySQL数据库来存储文件路径、文件名和文件内容。在搜索时,我们使用`LIKE`子句来匹配搜索路径和关键字。然后,我们将结果显示在一个JTable中。注意,这个示例只是一个简单的演示,你需要根据自己的需求进行修改和扩展。同时,你需要添加MySQL数据库的驱动程序到你的类路径中。
相关推荐
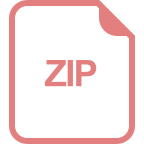














