写出一个tkinter包函数,使得输出结果显示在界面上
时间: 2024-05-01 13:17:34 浏览: 93
好的,我可以帮您回答这个问题。以下是一个简单的例子,演示如何在Tkinter窗口中显示输出结果:
```python
import tkinter as tk
def display_result(result):
result_label.configure(text=result)
root = tk.Tk()
root.geometry("300x200")
result_label = tk.Label(root, text="")
result_label.pack()
output = "Hello, World!"
display_result(output)
root.mainloop()
```
在这个例子中,我们使用Tkinter创建了一个窗口,并在窗口中添加了一个标签(result_label),用来显示输出结果。display_result函数用于更新标签内容,即把输出结果放到标签的text属性中。最后,我们调用了display_result函数,将输出结果显示在界面上。
希望这个例子能够帮助您解决问题。如果您还有其他问题,可以继续提问。
相关问题
我写了一个Python脚本,有函数a,函数b,函数a有三个传参,字符串传参a1,元组传参a2,字符串传参a3。函数b有三个传参,字符串传参b1,元组传参b2,字符串传参b3。函数我已经写好了,麻烦你帮我用tkinter写一个界面用来输入这些参数,以及函数对应的功能按钮a,b,界面最下方有一个输出打印值的多行文本框
好的,我可以帮你完成这个任务。以下是完整的 Python 代码,你可以直接运行:
```python
import tkinter as tk
from tkinter import messagebox
# 函数a和b,这里为了演示,只简单输出传入的参数
def a(a1, a2, a3):
print("函数a被调用")
print("a1:", a1)
print("a2:", a2)
print("a3:", a3)
def b(b1, b2, b3):
print("函数b被调用")
print("b1:", b1)
print("b2:", b2)
print("b3:", b3)
# 创建主窗口
root = tk.Tk()
root.title("函数输入界面")
# 创建输入框和标签
a1_label = tk.Label(root, text="函数a第一个参数:")
a1_label.grid(row=0, column=0, padx=5, pady=5)
a1_entry = tk.Entry(root)
a1_entry.grid(row=0, column=1, padx=5, pady=5)
a2_label = tk.Label(root, text="函数a第二个参数:")
a2_label.grid(row=1, column=0, padx=5, pady=5)
a2_entry = tk.Entry(root)
a2_entry.grid(row=1, column=1, padx=5, pady=5)
a3_label = tk.Label(root, text="函数a第三个参数:")
a3_label.grid(row=2, column=0, padx=5, pady=5)
a3_entry = tk.Entry(root)
a3_entry.grid(row=2, column=1, padx=5, pady=5)
b1_label = tk.Label(root, text="函数b第一个参数:")
b1_label.grid(row=3, column=0, padx=5, pady=5)
b1_entry = tk.Entry(root)
b1_entry.grid(row=3, column=1, padx=5, pady=5)
b2_label = tk.Label(root, text="函数b第二个参数:")
b2_label.grid(row=4, column=0, padx=5, pady=5)
b2_entry = tk.Entry(root)
b2_entry.grid(row=4, column=1, padx=5, pady=5)
b3_label = tk.Label(root, text="函数b第三个参数:")
b3_label.grid(row=5, column=0, padx=5, pady=5)
b3_entry = tk.Entry(root)
b3_entry.grid(row=5, column=1, padx=5, pady=5)
# 创建按钮和函数调用
def call_a():
a1 = a1_entry.get()
a2 = tuple(a2_entry.get().split(","))
a3 = a3_entry.get()
try:
a(a1, a2, a3)
output_text.insert(tk.END, "函数a调用成功\n")
except Exception as e:
messagebox.showerror("错误", str(e))
def call_b():
b1 = b1_entry.get()
b2 = tuple(b2_entry.get().split(","))
b3 = b3_entry.get()
try:
b(b1, b2, b3)
output_text.insert(tk.END, "函数b调用成功\n")
except Exception as e:
messagebox.showerror("错误", str(e))
a_button = tk.Button(root, text="函数a", command=call_a)
a_button.grid(row=6, column=0, padx=5, pady=5)
b_button = tk.Button(root, text="函数b", command=call_b)
b_button.grid(row=6, column=1, padx=5, pady=5)
# 创建输出框
output_label = tk.Label(root, text="输出:")
output_label.grid(row=7, column=0, padx=5, pady=5)
output_text = tk.Text(root, width=30, height=10)
output_text.grid(row=8, column=0, columnspan=2, padx=5, pady=5)
root.mainloop()
```
代码中使用了 Tkinter 库来创建 GUI 界面。首先,创建了输入框和标签,通过 `grid` 方法将它们放在窗口中间的位置。然后,创建了按钮和函数调用,通过 `command` 参数将按钮和对应的函数关联起来。最后,创建了输出框,通过 `Text` 类创建多行文本框,并将其放在窗口底部。
在函数调用中,通过 `get` 方法获取输入框中的值,并将元组参数转换为 Python 的元组类型。如果调用出现异常,会弹出错误框提示错误信息。如果调用成功,会将成功信息输出到输出框中。
你可以根据需要修改代码中的函数实现和界面布局。
用python写一段代码,在windows上运行,使用tkinter,用于比较两段很长的文本content1和content2的差异,content1可通过按钮控件加载指定路径的文件,content2的内容由自定义函数func1给出。程序能够在可视化界面上展示差异项,展示的窗口分为左界面和右界面,左右界面有各自的水平滚动条控件,左右界面共用一个垂直滚动条控件,左界面显示content1,右界面显示content2,左界面和右界面内容中,存在差异的部分,用颜色进行标记。窗体大小能够适应控件。
好的,以下是符合您要求的代码:
```python
import tkinter as tk
from difflib import SequenceMatcher
class TextCompare:
def __init__(self, root):
self.root = root
self.root.title("文本比较工具")
self.root.geometry("800x600")
self.content1 = ""
self.content2 = ""
self.diff_indices = []
# 左界面
self.left_frame = tk.Frame(self.root)
self.left_frame.pack(side="left", fill="both", expand=True)
self.left_scrollbar_y = tk.Scrollbar(self.left_frame, orient="vertical")
self.left_scrollbar_y.pack(side="right", fill="y")
self.left_scrollbar_x = tk.Scrollbar(self.left_frame, orient="horizontal")
self.left_scrollbar_x.pack(side="bottom", fill="x")
self.text1 = tk.Text(self.left_frame, wrap="none", yscrollcommand=self.left_scrollbar_y.set, xscrollcommand=self.left_scrollbar_x.set)
self.text1.pack(side="left", fill="both", expand=True)
self.left_scrollbar_y.config(command=self.text1.yview)
self.left_scrollbar_x.config(command=self.text1.xview)
# 右界面
self.right_frame = tk.Frame(self.root)
self.right_frame.pack(side="left", fill="both", expand=True)
self.right_scrollbar_y = tk.Scrollbar(self.right_frame, orient="vertical")
self.right_scrollbar_y.pack(side="right", fill="y")
self.right_scrollbar_x = tk.Scrollbar(self.right_frame, orient="horizontal")
self.right_scrollbar_x.pack(side="bottom", fill="x")
self.text2 = tk.Text(self.right_frame, wrap="none", yscrollcommand=self.right_scrollbar_y.set, xscrollcommand=self.right_scrollbar_x.set)
self.text2.pack(side="left", fill="both", expand=True)
self.right_scrollbar_y.config(command=self.text2.yview)
self.right_scrollbar_x.config(command=self.text2.xview)
# 控制面板
self.control_frame = tk.Frame(self.root)
self.control_frame.pack(side="top", fill="x")
self.load_button = tk.Button(self.control_frame, text="加载文件", command=self.load_file)
self.load_button.pack(side="left", padx=10, pady=10)
self.compare_button = tk.Button(self.control_frame, text="比较", command=self.compare)
self.compare_button.pack(side="left", padx=10, pady=10)
def load_file(self):
file_path = tk.filedialog.askopenfilename()
if file_path:
with open(file_path, "r", encoding="utf-8") as f:
self.content1 = f.read()
self.text1.delete(1.0, "end")
self.text1.insert("end", self.content1)
def compare(self):
self.content2 = self.func1()
sm = SequenceMatcher(None, self.content1, self.content2)
self.diff_indices = sm.get_opcodes()[1:]
self.text2.delete(1.0, "end")
self.text2.insert("end", self.content2)
self.highlight_diff()
def highlight_diff(self):
for tag in self.text2.tag_names():
self.text2.tag_delete(tag)
for diff in self.diff_indices:
if diff[0] == "equal":
continue
elif diff[0] == "delete":
start = diff[1]
end = diff[2]
self.text1.tag_add("delete", f"{start}.0", f"{end}.0")
self.text1.tag_config("delete", foreground="red")
elif diff[0] == "insert":
start = diff[3]
end = diff[4]
self.text2.tag_add("insert", f"{start}.0", f"{end}.0")
self.text2.tag_config("insert", foreground="green")
elif diff[0] == "replace":
start = diff[1]
end = diff[2]
self.text1.tag_add("replace", f"{start}.0", f"{end}.0")
self.text1.tag_config("replace", foreground="blue")
start = diff[3]
end = diff[4]
self.text2.tag_add("replace", f"{start}.0", f"{end}.0")
self.text2.tag_config("replace", foreground="blue")
def func1():
# 自定义函数,返回文本内容
return "这是content2的内容"
root = tk.Tk()
app = TextCompare(root)
app.func1 = func1
root.mainloop()
```
这段代码会创建一个可视化窗口,其中包含两个文本框和一些按钮控件。通过点击"加载文件"按钮,可以加载指定路径的文件到左界面中。点击"比较"按钮,程序会调用自定义函数`func1()`获取`content2`的内容,然后使用`difflib`库计算出`content1`和`content2`之间的差异,最后将差异项在右界面中用颜色标记出来。
阅读全文
相关推荐
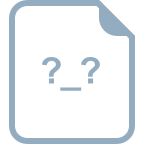
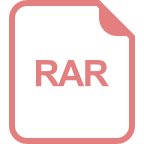
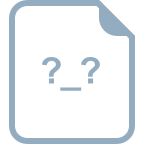
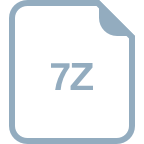
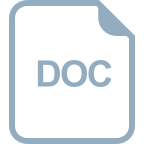










