Pinia如何进行异步操纵
时间: 2024-03-23 21:38:11 浏览: 11
在 Pinia 中,异步操作可以通过使用 actions 来实现。Actions 是 store 中的方法,可以进行异步操作,例如发起 API 请求等。在 actions 中,你可以通过 `await` 关键字来等待异步操作的完成,并使用 `commit` 函数来提交 mutation,从而修改 store 中的状态。
下面是一个示例,假设我们有一个名为 counter 的 store,其中包含了一个名为 count 的状态属性,我们想要定义一个名为 `incrementAsync` 的 action 函数,该函数将在 1 秒后将 count 值增加 1:
```js
import { defineStore } from 'pinia'
export const useCounterStore = defineStore({
id: 'counter',
state: () => ({
count: 0,
}),
actions: {
async incrementAsync() {
await new Promise(resolve => setTimeout(resolve, 1000))
this.count++
},
},
})
```
在组件中,我们可以通过 `useStore` 函数来获取 store,然后调用其 action 函数来执行异步操作,如下所示:
```vue
<template>
<div>
<p>Count: {{ count }}</p>
<button @click="incrementAsync">Increment Async</button>
</div>
</template>
<script>
import { useCounterStore } from './store'
export default {
setup() {
const store = useCounterStore()
async function incrementAsync() {
await store.incrementAsync()
}
return {
count: store.count,
incrementAsync,
}
},
}
</script>
```
在这个例子中,我们在 setup 函数中通过 `useCounterStore` 获取了 counter store,并将其分配给变量 `store`。然后我们定义了一个 `incrementAsync` 函数,该函数通过 `await store.incrementAsync()` 来调用 `incrementAsync` action,等待异步操作完成后更新 count 的值。最后,我们将 count 的值和 incrementAsync 函数返回给模板,以便能够在模板中显示 count 的值,并在按钮上绑定 incrementAsync 函数。
相关推荐
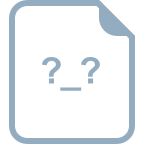














