使用 Java类写一个蓝牙测试代码,用于搜索和显示蓝牙状态,并利用view显示出来,类名为BluetoothTest
时间: 2024-02-24 10:57:51 浏览: 135
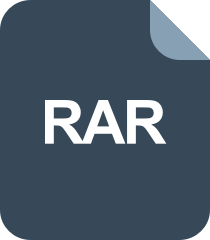
蓝牙测试程序
好的,下面是使用 Java 类编写的蓝牙测试代码,用于搜索和显示蓝牙状态,并利用 View 显示出来,类名为 BluetoothTest:
```java
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.content.Context;
import android.support.annotation.Nullable;
import android.util.AttributeSet;
import android.view.View;
import android.widget.LinearLayout;
import android.widget.TextView;
import java.util.Set;
public class BluetoothTest extends LinearLayout {
private BluetoothAdapter mBluetoothAdapter;
private TextView mBluetoothStatus;
private TextView mPairedDevices;
public BluetoothTest(Context context) {
super(context);
init();
}
public BluetoothTest(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
init();
}
public BluetoothTest(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
private void init() {
View rootView = inflate(getContext(), R.layout.bluetooth_test, this);
mBluetoothStatus = rootView.findViewById(R.id.bluetooth_status);
mPairedDevices = rootView.findViewById(R.id.paired_devices);
mBluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
}
public void searchDevices() {
mBluetoothStatus.setText("Searching...");
Set<BluetoothDevice> pairedDevices = mBluetoothAdapter.getBondedDevices();
if (pairedDevices.size() > 0) {
StringBuilder devices = new StringBuilder();
for (BluetoothDevice device : pairedDevices) {
devices.append(device.getName()).append("\n");
}
mPairedDevices.setText(devices.toString());
} else {
mPairedDevices.setText("No paired devices found.");
}
mBluetoothStatus.setText("Search finished.");
}
}
```
代码说明:
1. `BluetoothTest` 继承自 LinearLayout,用于将 View 显示出来;
2. `mBluetoothAdapter` 是 BluetoothAdapter 对象,用于操作 Bluetooth;
3. `mBluetoothStatus` 和 `mPairedDevices` 是两个 TextView,用于显示蓝牙状态和已配对设备;
4. `init()` 方法用于初始化 View;
5. `searchDevices()` 方法用于搜索已配对的蓝牙设备并将其显示在 mPairedDevices 中。
需要注意的是,由于蓝牙操作需要权限,需要在 AndroidManifest.xml 中添加以下代码:
```xml
<uses-permission android:name="android.permission.BLUETOOTH" />
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN" />
```
阅读全文
相关推荐

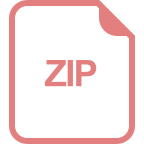
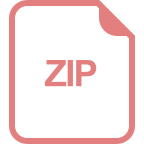
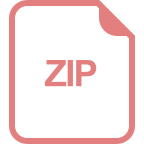
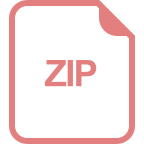
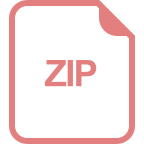
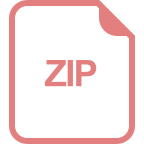
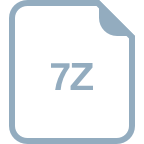
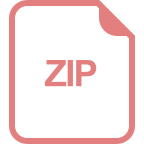
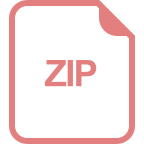
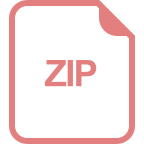
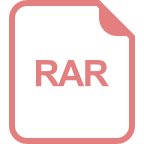
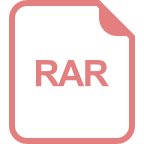
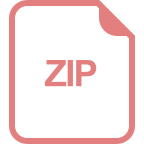
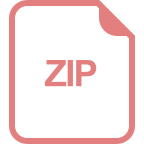
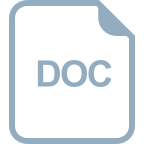