用c++写一个汽车租赁系统
时间: 2024-02-18 07:34:29 浏览: 33
好的,下面是一个简单的汽车租赁系统的示例代码,仅供参考:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Car {
public:
string brand;
string model;
int year;
double price;
bool available;
Car(string b, string m, int y, double p, bool a) {
brand = b;
model = m;
year = y;
price = p;
available = a;
}
};
class Rental {
public:
string renter_name;
Car car;
int rental_days;
Rental(string rn, Car c, int rd) {
renter_name = rn;
car = c;
rental_days = rd;
}
double get_total_price() {
return rental_days * car.price;
}
};
int main() {
vector<Car> cars;
cars.push_back(Car("Toyota", "Camry", 2015, 50.0, true));
cars.push_back(Car("Honda", "Accord", 2018, 70.0, true));
cars.push_back(Car("Ford", "Mustang", 2020, 120.0, true));
vector<Rental> rentals;
while (true) {
cout << "1. Rent a car" << endl;
cout << "2. Return a car" << endl;
cout << "3. Exit" << endl;
cout << "Enter your choice: ";
int choice;
cin >> choice;
if (choice == 1) {
cout << "Available cars:" << endl;
for (int i = 0; i < cars.size(); i++) {
if (cars[i].available) {
cout << i << ". " << cars[i].brand << " " << cars[i].model << " (" << cars[i].year << ") - $" << cars[i].price << "/day" << endl;
}
}
int car_choice;
cout << "Enter car choice: ";
cin >> car_choice;
if (cars[car_choice].available) {
string renter_name;
cout << "Enter renter name: ";
cin >> renter_name;
int rental_days;
cout << "Enter rental days: ";
cin >> rental_days;
cars[car_choice].available = false;
rentals.push_back(Rental(renter_name, cars[car_choice], rental_days));
cout << "Successfully rented " << cars[car_choice].brand << " " << cars[car_choice].model << " to " << renter_name << "." << endl;
} else {
cout << "Car is not available." << endl;
}
} else if (choice == 2) {
cout << "Rented cars:" << endl;
for (int i = 0; i < rentals.size(); i++) {
cout << i << ". " << rentals[i].car.brand << " " << rentals[i].car.model << " (" << rentals[i].car.year << ") - $" << rentals[i].car.price << "/day - Rented to " << rentals[i].renter_name << " for " << rentals[i].rental_days << " days." << endl;
}
int rental_choice;
cout << "Enter rental choice: ";
cin >> rental_choice;
if (rental_choice < rentals.size()) {
double total_price = rentals[rental_choice].get_total_price();
cout << "Total price for " << rentals[rental_choice].rental_days << " days is $" << total_price << "." << endl;
cars.push_back(rentals[rental_choice].car);
cars.back().available = true;
rentals.erase(rentals.begin() + rental_choice);
cout << "Successfully returned " << cars.back().brand << " " << cars.back().model << "." << endl;
} else {
cout << "Invalid rental choice." << endl;
}
} else if (choice == 3) {
break;
} else {
cout << "Invalid choice." << endl;
}
}
return 0;
}
```
这个系统使用了两个类:`Car` 和 `Rental`,分别表示汽车和租赁情况。主函数中创建了一个汽车列表和一个租赁列表,然后进入一个循环,让用户进行操作。
用户可以选择租用汽车或归还汽车,当租用汽车时,系统会列出当前可用的汽车列表,并要求用户输入租用的汽车、租用天数和租用人的姓名。当归还汽车时,系统会列出当前已租用的汽车列表,并要求用户输入要归还的租赁记录。系统会计算出租赁总价,并将汽车标记为可用状态,从租赁列表中删除相应的记录。
这只是一个简单的示例代码,实际的汽车租赁系统可能需要更复杂的功能和更完善的界面设计。
相关推荐
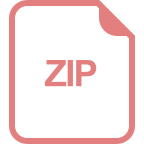
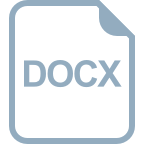
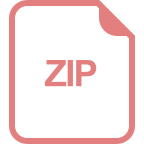









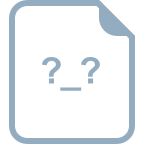
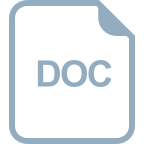
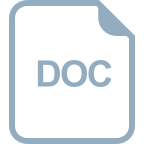
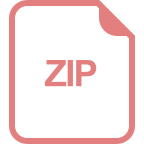
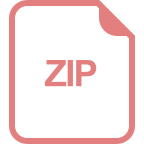
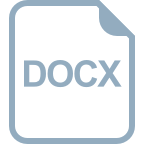