调用本地图片进行CNN模型识别
时间: 2023-05-30 12:04:39 浏览: 209
首先,需要将本地图片读取到内存中。可以使用Python的Pillow库来读取和处理图片。
```python
from PIL import Image
# 读取图片
img = Image.open('path/to/image.jpg')
# 转换为RGB格式
img = img.convert('RGB')
# 调整大小为模型输入的大小
img = img.resize((224, 224))
```
接下来,需要将图片转换为模型需要的格式。一般来说,CNN模型需要输入为一个4维张量,其形状为`(batch_size, height, width, channels)`,其中`batch_size`表示批量大小,`height`和`width`表示图片的高度和宽度,`channels`表示图片的通道数。
对于一张图片,可以将`batch_size`设置为1,`height`和`width`设置为模型输入的大小(比如224),`channels`设置为3,即RGB三个通道。
```python
import numpy as np
# 将图片转换为numpy数组
img_array = np.array(img)
# 将值缩放到0-1之间
img_array = img_array / 255.0
# 将数组转换为4维张量
img_tensor = np.expand_dims(img_array, axis=0)
```
最后,可以调用CNN模型进行识别。使用TensorFlow的Keras API可以方便地加载预训练模型,并进行预测。
```python
import tensorflow as tf
from tensorflow.keras.applications.resnet50 import ResNet50, preprocess_input, decode_predictions
# 加载预训练模型
model = ResNet50(weights='imagenet')
# 预处理输入
img_tensor = preprocess_input(img_tensor)
# 进行预测
preds = model.predict(img_tensor)
# 解码预测结果
decoded_preds = decode_predictions(preds, top=3)[0]
# 输出预测结果
for pred in decoded_preds:
print(pred[1], pred[2])
```
其中,`decode_predictions`函数将模型输出的概率向量解码为具体的类别标签和对应的置信度。`top`参数表示输出置信度最高的几个类别。
阅读全文
相关推荐


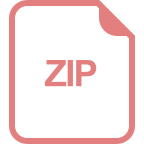
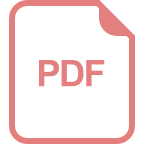
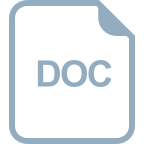
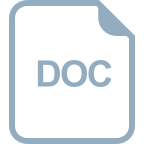
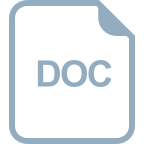
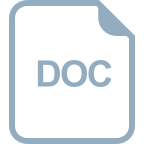
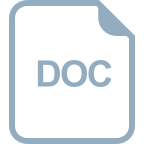
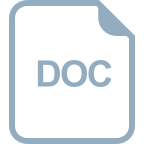
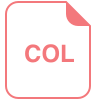





