如何给函数返回增加缓存
时间: 2024-04-27 10:24:10 浏览: 75
可以使用 memoization 技术来给函数返回增加缓存。这种技术可以将函数的输入和输出缓存起来,在下次函数被调用时,如果输入值已经被缓存了,就可以直接返回缓存中的输出值,从而避免了重复计算。具体实现可以通过使用哈希表等数据结构来存储输入和输出值的映射关系,并在每次函数被调用时查询缓存,如果存在缓存则直接返回缓存中的值,否则计算函数输出并将输入和输出存入缓存中。这种技术可以大大提升函数的执行效率,特别是对于一些耗时的计算任务。
相关问题
java 如何给函数返回增加缓存
在 Java 中,可以使用 HashMap 来实现函数的缓存。具体实现步骤如下:
1. 创建一个 HashMap 对象,用于存储函数的输入和输出值。
```java
private static Map<Integer, Integer> cache = new HashMap<>();
```
2. 修改函数的实现,加入缓存逻辑,先从缓存中查找输入值对应的输出值,如果存在则直接返回,否则进行计算,并将计算结果存入缓存中。
```java
public static int fibonacci(int n) {
if (n < 0) {
throw new IllegalArgumentException("n must be greater than or equal to zero");
}
if (n == 0 || n == 1) {
return n;
}
if (cache.containsKey(n)) {
return cache.get(n);
}
int result = fibonacci(n - 1) + fibonacci(n - 2);
cache.put(n, result);
return result;
}
```
这样,每次调用函数时,先查询缓存,如果存在则直接返回缓存中的结果,否则进行计算,并将结果存入缓存中。这样可以避免重复计算,提高函数执行效率。需要注意的是,缓存的存储空间是有限的,如果缓存中的数据量过大,可能会导致内存溢出等问题,因此需要根据具体情况进行合理的缓存策略。
spring 如何给函数返回增加缓存
在 Spring 中,可以使用 Spring Cache 技术来实现函数的缓存。具体实现步骤如下:
1. 在 pom.xml 文件中添加依赖。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
```
2. 在 Spring Boot 应用的启动类上添加 @EnableCaching 注解,开启缓存功能。
```java
@SpringBootApplication
@EnableCaching
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
3. 在需要缓存的函数上添加 @Cacheable 注解,指定缓存的名称和缓存的 Key。
```java
@Service
public class UserService {
@Autowired
private UserDao userDao;
@Cacheable(value = "userCache", key = "#id")
public User getUserById(int id) {
return userDao.getUserById(id);
}
}
```
4. 配置缓存管理器,可以使用 Ehcache、Redis 等缓存实现,也可以使用 Spring Boot 默认的 ConcurrentMapCacheManager。在 application.properties 文件中添加如下配置:
```properties
spring.cache.type=redis
spring.redis.host=localhost
spring.redis.port=6379
```
这样,每次调用 getUserById 方法时,会先从缓存中查找对应的数据,如果缓存中存在,则直接返回缓存中的数据;如果缓存中不存在,则调用 userDao.getUserById 方法获取数据,并将数据存入缓存中。需要注意的是,缓存的 Key 可以使用 SpEL 表达式来动态生成,例如可以使用 "#user.id" 表示以用户 id 作为缓存的 Key。另外,Spring Cache 还支持 @CachePut、@CacheEvict、@Caching 等注解,用于更新缓存、清除缓存等操作。
阅读全文
相关推荐
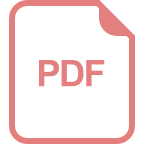
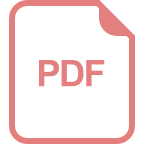
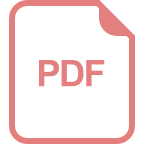
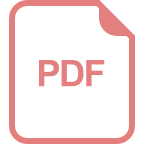
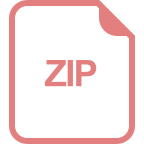
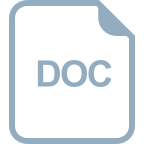
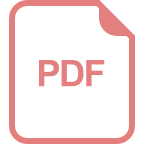
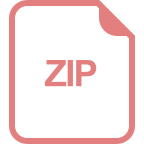
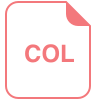
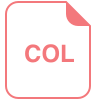
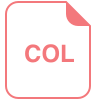
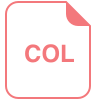
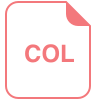
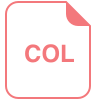
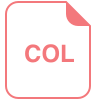
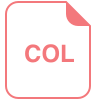
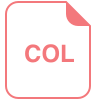
