orderInvoiceData.value.forEach(item => { item.invoiceList.forEach(i => { i.detail_list.forEach(index => { if (index.tax_code && index.tax_code.toString() !== '错误编码') { index.tax_code = index.tax_code.padEnd(codeLength, '0') } }) }) }) 优化这三层for循环的代码
时间: 2024-03-06 08:48:58 浏览: 19
可以使用 `Array.prototype.flatMap()` 方法和 `Array.prototype.map()` 方法来简化这段代码:
```
orderInvoiceData.value.flatMap(item => item.invoiceList)
.flatMap(i => i.detail_list)
.forEach(index => {
if (index.tax_code && index.tax_code.toString() !== '错误编码') {
index.tax_code = index.tax_code.padEnd(codeLength, '0')
}
});
```
这里使用了两个 `flatMap()` 方法,第一个将 `orderInvoiceData.value` 中的所有发票列表扁平化成一个数组,第二个将每个发票的详情列表扁平化成一个数组。最后通过 `forEach()` 方法遍历所有的详情列表,进行税号填充的操作。这种方式可以避免多层嵌套的循环,使代码更加简洁易懂。
相关问题
优化这段代码:private List<DynamicSource> getDynamicSourceList(List<DasymesQltyMpCertfInfoItemMod> itemModList) { List<Map<String, String>> mapList = new ArrayList<>(); if (itemModList == null || itemModList.size() == 0) { Map<String, String> map = new HashMap<>(); map.put("no", null); map.put("name", null); map.put("portion", null); mapList.add(map); return DynamicSource.createList("detail", mapList); } for (int i = 1; i <= itemModList.size(); i++) { DasymesQltyMpCertfInfoItemMod item = itemModList.get(i - 1); Map<String, String> map = new HashMap<>(); map.put("no", i + ""); map.put("name", item.getItemName()); map.put("portion", item.getPortion()); mapList.add(map); } return DynamicSource.createList("detail", mapList); }
可以优化的地方如下:
1. 在if语句中,可以直接创建map并添加到mapList中,避免了在后面的循环中再创建map。
2. 在循环中,可以使用foreach循环代替for循环,避免了使用i变量。
3. 在创建DynamicSource时,可以直接使用mapList,不需要再创建一个中间变量。
优化后的代码如下:
```
private List<DynamicSource> getDynamicSourceList(List<DasymesQltyMpCertfInfoItemMod> itemModList) {
List<Map<String, String>> mapList = new ArrayList<>();
if (itemModList == null || itemModList.isEmpty()) {
mapList.add(Map.of(
"no", null,
"name", null,
"portion", null
));
} else {
for (DasymesQltyMpCertfInfoItemMod item : itemModList) {
Map<String, String> map = new HashMap<>();
map.put("no", String.valueOf(mapList.size() + 1));
map.put("name", item.getItemName());
map.put("portion", item.getPortion());
mapList.add(map);
}
}
return DynamicSource.createList("detail", mapList);
}
```
这样代码更加简洁易读,也更加高效。
写微信小程序代码,功能如下 1、有一个模糊查询 2、有一个分页查询 3、有一个动态if 4、有一个动态where 5、有一个动态foreach 6、有一个动态片段1个 7、做多参数传递使用@Param一个 8、做多参数传递使用arg位置 9、做对象传递一个 10、使用$一个。每处代码写注释
好的,以下是一个简单的示例代码,主要是为了演示如何实现上述功能,具体的实现方式根据业务需求会有所不同。
1. 模糊查询
在 WXML 文件中添加一个输入框和搜索按钮:
```
<view class="search-box">
<input class="search-input" bindinput="onInput" placeholder="请输入搜索关键字"></input>
<button class="search-btn" bindtap="onSearch">搜索</button>
</view>
```
在 JS 文件中实现 onInput 和 onSearch 函数:
```
Page({
data: {
keyword: '', // 搜索关键字
list: [] // 搜索结果列表
},
// 输入搜索关键字
onInput(e) {
this.setData({
keyword: e.detail.value
})
},
// 搜索
onSearch() {
wx.request({
url: 'https://example.com/search',
data: {
keyword: this.data.keyword
},
success: res => {
this.setData({
list: res.data
})
}
})
}
})
```
2. 分页查询
在 WXML 文件中添加一个列表和分页组件:
```
<view class="list-box">
<block wx:for="{{list}}">
<view class="item">{{item.name}}</view>
</block>
</view>
<view class="page-box">
<page-navigator class="prev" previous="{{currentPage > 1}}" bindtap="onPagePrev">上一页</page-navigator>
<text class="page-info">{{currentPage}}/{{totalPage}}</text>
<page-navigator class="next" next="{{currentPage < totalPage}}" bindtap="onPageNext">下一页</page-navigator>
</view>
```
在 JS 文件中实现 onPagePrev 和 onPageNext 函数:
```
Page({
data: {
currentPage: 1, // 当前页码
totalPage: 1, // 总页数
list: [] // 列表数据
},
// 上一页
onPagePrev() {
if (this.data.currentPage > 1) {
this.loadData(this.data.currentPage - 1);
}
},
// 下一页
onPageNext() {
if (this.data.currentPage < this.data.totalPage) {
this.loadData(this.data.currentPage + 1);
}
},
// 加载数据
loadData(page) {
wx.request({
url: 'https://example.com/list',
data: {
page: page
},
success: res => {
this.setData({
currentPage: res.data.currentPage,
totalPage: res.data.totalPage,
list: res.data.list
})
}
})
},
// 页面加载时加载第一页数据
onLoad() {
this.loadData(1);
}
})
```
3. 动态 if
在 WXML 文件中添加一个条件渲染的元素:
```
<view wx:if="{{isShow}}">我是动态渲染的元素</view>
```
在 JS 文件中实现 isShow 变量的动态修改:
```
Page({
data: {
isShow: true
},
// 切换是否显示
toggleShow() {
this.setData({
isShow: !this.data.isShow
})
}
})
```
4. 动态 where
在后端接口实现动态拼接 SQL 查询语句,例如:
```
select * from table where 1=1
<if test="param1 != null">
and field1 like concat('%', #{param1}, '%')
</if>
<if test="param2 != null">
and field2 = #{param2}
</if>
```
5. 动态 foreach
在 WXML 文件中使用 block 标签和 wx:for 属性渲染列表:
```
<block wx:for="{{list}}">
<view>{{item.name}}</view>
</block>
```
在 JS 文件中动态传递数据给 list 变量:
```
Page({
data: {
list: []
},
// 加载数据
loadData() {
wx.request({
url: 'https://example.com/list',
success: res => {
this.setData({
list: res.data
})
}
})
},
// 页面加载时加载数据
onLoad() {
this.loadData();
}
})
```
6. 动态片段
在 WXML 文件中使用 template 标签定义一个动态片段:
```
<template name="myTemplate">
<view>{{text}}</view>
</template>
```
在需要使用该片段的地方使用 include 标签引用:
```
<include src="myTemplate"></include>
```
7. 多参数传递
在 WXML 文件中使用 @Param 注解和 arg 参数传递参数:
```
<navigator url="/pages/detail/detail?@Param={{paramObj}}&arg1={{arg1}}&arg2={{arg2}}">跳转到详情页</navigator>
```
在详情页的 JS 文件中使用 onLoad 函数获取参数:
```
Page({
onLoad(options) {
const { paramObj } = options.@Param;
const { arg1, arg2 } = options;
}
})
```
8. 多参数传递
在 WXML 文件中使用 arg 参数传递参数:
```
<navigator url="/pages/detail/detail?arg={{argObj}}">跳转到详情页</navigator>
```
在详情页的 JS 文件中使用 onLoad 函数获取参数:
```
Page({
onLoad(options) {
const { argObj } = options;
}
})
```
9. 对象传递
直接将对象作为参数传递给后台接口或小程序端:
```
wx.request({
url: 'https://example.com/submit',
data: {
name: '张三',
age: 18
},
success: res => {
console.log(res.data);
}
})
```
10. 使用 $
在 WXML 中使用 $ 绑定数据或事件:
```
<view>{{ $data.text }}</view>
<view bindtap="$handleTap"></view>
```
在 JS 中使用 $ 获取元素:
```
const query = wx.createSelectorQuery();
query.select('#myId').boundingClientRect(function(rect) {
console.log(rect.width);
}).exec();
```
以上是一个简单的示例代码,具体实现方式和业务需求可能有所不同,但是思路和方法是相通的。
相关推荐
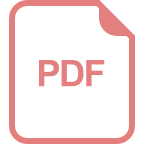








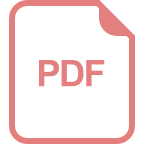