使用Python采用数据库存储数据做一个学校教材管理系统实现增删查改以及排序、统计并且交互式界面设计直观体现系统功能并可循环调用
时间: 2024-01-29 21:02:24 浏览: 176
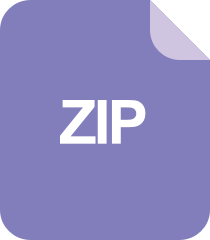
基于python语言开发的高校教材管理系统

好的,以下是一个使用Python和SQLite实现增删查改、排序、统计以及交互式界面设计的学校教材管理系统示例。
#### 1. 安装必要的库
```python
pip install sqlite3
pip install prettytable
pip install PySimpleGUI
```
#### 2. 创建数据库和表
我们将创建一个名为 `textbooks.db` 的SQLite数据库,并在其中创建一个名为 `textbooks` 的表。
```python
import sqlite3
# 连接到数据库并创建一个游标
conn = sqlite3.connect('textbooks.db')
c = conn.cursor()
# 创建表
c.execute('CREATE TABLE IF NOT EXISTS textbooks (id INTEGER PRIMARY KEY, title TEXT, author TEXT, publisher TEXT, year INTEGER)')
# 提交更改并关闭连接
conn.commit()
conn.close()
```
#### 3. 实现增删查改功能
```python
import sqlite3
# 连接到数据库并创建一个游标
conn = sqlite3.connect('textbooks.db')
c = conn.cursor()
# 添加教材
def add_textbook(title, author, publisher, year):
c.execute('INSERT INTO textbooks (title, author, publisher, year) VALUES (?, ?, ?, ?)', (title, author, publisher, year))
conn.commit()
# 删除教材
def delete_textbook(id):
c.execute('DELETE FROM textbooks WHERE id = ?', (id,))
conn.commit()
# 搜索教材
def search_textbook(title):
c.execute('SELECT * FROM textbooks WHERE title LIKE ?', ('%' + title + '%',))
return c.fetchall()
# 更新教材
def update_textbook(id, title, author, publisher, year):
c.execute('UPDATE textbooks SET title = ?, author = ?, publisher = ?, year = ? WHERE id = ?', (title, author, publisher, year, id))
conn.commit()
# 关闭连接
conn.close()
```
#### 4. 实现排序和统计功能
```python
import sqlite3
from prettytable import PrettyTable
# 连接到数据库并创建一个游标
conn = sqlite3.connect('textbooks.db')
c = conn.cursor()
# 获取所有教材
def get_all_textbooks():
c.execute('SELECT * FROM textbooks')
return c.fetchall()
# 按标题排序
def sort_by_title():
c.execute('SELECT * FROM textbooks ORDER BY title')
return c.fetchall()
# 按出版年份排序
def sort_by_year():
c.execute('SELECT * FROM textbooks ORDER BY year')
return c.fetchall()
# 统计教材数量
def count_textbooks():
c.execute('SELECT COUNT(*) FROM textbooks')
return c.fetchone()[0]
# 关闭连接
conn.close()
```
#### 5. 实现交互式界面
我们将使用 PySimpleGUI 库来创建一个简单的GUI,以便用户可以轻松地使用学校教材管理系统。
```python
import PySimpleGUI as sg
# 定义GUI布局
layout = [
[sg.Text('学校教材管理系统', font=('Arial', 20), justification='center', size=(30, 1))],
[sg.Text('标题:'), sg.InputText(key='title')],
[sg.Text('作者:'), sg.InputText(key='author')],
[sg.Text('出版社:'), sg.InputText(key='publisher')],
[sg.Text('出版年份:'), sg.InputText(key='year')],
[sg.Button('添加', key='add'), sg.Button('删除', key='delete'), sg.Button('搜索', key='search'), sg.Button('更新', key='update')],
[sg.Button('按标题排序', key='sort_by_title'), sg.Button('按出版年份排序', key='sort_by_year'), sg.Button('统计教材数量', key='count')],
[sg.Table(values=[], headings=['ID', '标题', '作者', '出版社', '出版年份'], key='table')],
[sg.Text('操作结果:'), sg.Text('', key='result', size=(50, 1))]
]
# 创建GUI窗口
window = sg.Window('学校教材管理系统', layout)
# 消息循环
while True:
event, values = window.Read()
# 添加教材
if event == 'add':
if values['title'] == '' or values['author'] == '' or values['publisher'] == '' or values['year'] == '':
window.Element('result').Update('请输入完整的教材信息!')
else:
add_textbook(values['title'], values['author'], values['publisher'], values['year'])
window.Element('result').Update('添加成功!')
# 删除教材
elif event == 'delete':
selected_rows = window.Element('table').GetSelectedRows()
if len(selected_rows) == 0:
window.Element('result').Update('请先选择要删除的教材!')
else:
for row in selected_rows:
delete_textbook(window.Element('table').GetCellValue(row, 0))
window.Element('result').Update('删除成功!')
# 搜索教材
elif event == 'search':
if values['title'] == '':
window.Element('result').Update('请输入要搜索的教材标题!')
else:
textbooks = search_textbook(values['title'])
if len(textbooks) == 0:
window.Element('result').Update('没有找到相关教材!')
else:
table_data = [[str(textbook[0]), textbook[1], textbook[2], textbook[3], str(textbook[4])] for textbook in textbooks]
window.Element('table').Update(values=table_data)
window.Element('result').Update('搜索成功!')
# 更新教材
elif event == 'update':
selected_rows = window.Element('table').GetSelectedRows()
if len(selected_rows) == 0:
window.Element('result').Update('请先选择要更新的教材!')
elif len(selected_rows) > 1:
window.Element('result').Update('一次只能更新一本教材!')
else:
row = selected_rows[0]
update_textbook(window.Element('table').GetCellValue(row, 0), values['title'], values['author'], values['publisher'], values['year'])
window.Element('result').Update('更新成功!')
# 按标题排序
elif event == 'sort_by_title':
textbooks = sort_by_title()
table_data = [[str(textbook[0]), textbook[1], textbook[2], textbook[3], str(textbook[4])] for textbook in textbooks]
window.Element('table').Update(values=table_data)
window.Element('result').Update('按标题排序成功!')
# 按出版年份排序
elif event == 'sort_by_year':
textbooks = sort_by_year()
table_data = [[str(textbook[0]), textbook[1], textbook[2], textbook[3], str(textbook[4])] for textbook in textbooks]
window.Element('table').Update(values=table_data)
window.Element('result').Update('按出版年份排序成功!')
# 统计教材数量
elif event == 'count':
count = count_textbooks()
window.Element('result').Update(f'教材数量为:{count}本')
# 关闭窗口
elif event == sg.WIN_CLOSED:
break
# 关闭窗口
window.Close()
```
运行上述代码,您将看到一个交互式的GUI窗口,您可以使用它来添加、删除、搜索、更新、排序和统计教材。
阅读全文
相关推荐
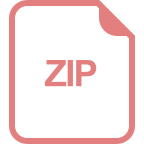
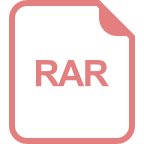

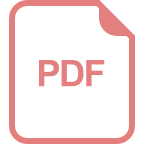
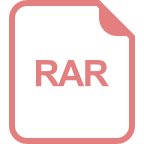
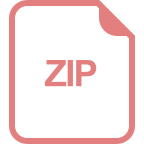
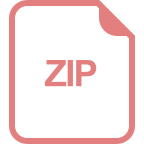
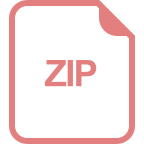
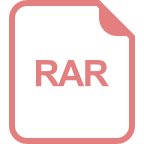
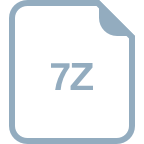
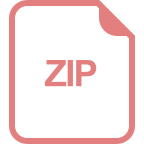
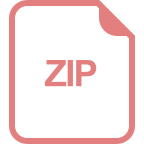
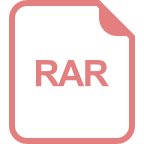
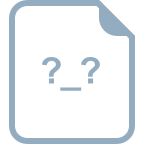
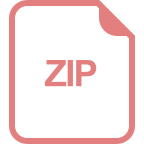
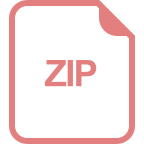
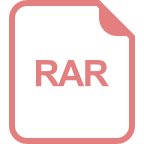