Socket通信c#样例程序
时间: 2023-05-24 22:05:12 浏览: 75
Socket通信是一种用于实现网络通信的编程接口,C语言中有很多库可以用来实现Socket通信,比如BSD Socket和Winsock等。在C语言中,可以使用Socket API中提供的函数来创建、连接、发送和接收数据。要使用Socket通信,需要首先创建一个Socket,然后将其绑定到本地IP地址和端口号上,接着可以使用该Socket来连接到远程主机或接受远程主机的连接,并通过发送和接收数据来实现通信。需要注意的是,Socket通信需要使用协议来规定通信的格式和内容,常用的协议有TCP和UDP。
相关问题
给一段C# TCP 通讯样例
在C#中,TCP通信通常通过System.Net.Sockets命名空间下的Socket类来实现。下面是一个简单的服务器和客户端的TCP通信示例:
**服务器端代码(Server.cs):**
```csharp
using System;
using System.Net;
using System.Net.Sockets;
class Server
{
private static TcpListener listener;
public static void Main()
{
// 创建监听器,指定端口
listener = new TcpListener(IPAddress.Any, 8080);
try
{
// 开始监听连接
listener.Start();
Console.WriteLine("Server started. Listening on port 8080...");
// 等待客户端连接
Socket client = listener.AcceptSocket();
// 接收客户端数据并处理
byte[] buffer = new byte[1024];
int bytesRead = client.Receive(buffer);
string receivedMessage = Encoding.ASCII.GetString(buffer, 0, bytesRead);
Console.WriteLine($"Received message from client: {receivedMessage}");
// 发送响应给客户端
string response = "Hello, Client!";
client.Send(Encoding.ASCII.GetBytes(response));
// 关闭连接
client.Close();
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
}
```
**客户端代码(Client.cs):**
```csharp
using System;
using System.Net.Sockets;
using System.Text;
class Client
{
static void Main()
{
// 连接到服务器地址和端口
TcpClient client = new TcpClient("127.0.0.1", 8080);
try
{
// 获取套接字流
NetworkStream stream = client.GetStream();
// 发送消息到服务器
string message = "Hello, Server!";
byte[] bytesToWrite = Encoding.ASCII.GetBytes(message);
stream.Write(bytesToWrite, 0, bytesToWrite.Length);
// 读取服务器响应
byte[] buffer = new byte[1024];
int bytesRead = stream.Read(buffer, 0, buffer.Length);
string response = Encoding.ASCII.GetString(buffer, 0, bytesRead);
Console.WriteLine($"Response from server: {response}");
// 关闭套接字
client.Close();
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
}
```
运行上述代码后,服务器会等待客户端连接,然后可以互相发送消息。请注意在实际应用中,你可能需要添加异常处理和更复杂的错误检查。
阅读全文
相关推荐






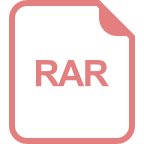



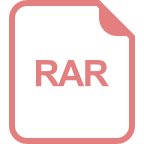
