winform自定义控件编写
时间: 2023-09-30 22:06:27 浏览: 171
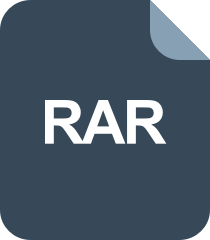
winform自定义控件
WinForm 是微软提供的用于创建 Windows 应用程序的框架,它提供了许多常用的控件,如按钮、标签、文本框等。但是,在实际开发中,我们可能需要自己编写一些特定的控件来实现一些特殊的功能。这时,我们就需要自定义控件。
以下是自定义 WinForm 控件的步骤:
1.创建一个类库项目
首先,我们需要创建一个类库项目,用于存放我们自定义的控件类。在 Visual Studio 中,可以通过“文件”->“新建”->“项目”->“类库”来创建。
2.添加引用
在类库项目中,我们需要添加对 System.Windows.Forms 的引用,这样才能够使用 WinForm 控件。
3.创建控件类
在类库项目中,我们可以创建一个控件类,该类继承自 System.Windows.Forms.Control。
例如,我们要编写一个自定义的按钮控件,可以创建一个类 ButtonEx,如下所示:
```csharp
public class ButtonEx : Control
{
//控件的状态
private bool _isMouseOver = false;
private bool _isMouseDown = false;
public ButtonEx()
{
//设置控件的基本属性
this.SetStyle(ControlStyles.StandardClick | ControlStyles.StandardDoubleClick, true);
this.SetStyle(ControlStyles.SupportsTransparentBackColor, true);
this.DoubleBuffered = true;
this.BackColor = Color.Transparent;
this.ForeColor = Color.Black;
this.Font = new Font("宋体", 9f, FontStyle.Regular, GraphicsUnit.Point, ((byte)(134)));
this.Size = new Size(75, 23);
}
//重写基类的 OnPaint 方法,绘制控件
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
//绘制按钮的背景和边框
if (_isMouseDown)
{
e.Graphics.FillRectangle(Brushes.LightGray, this.ClientRectangle);
}
else if (_isMouseOver)
{
e.Graphics.FillRectangle(Brushes.Gray, this.ClientRectangle);
}
else
{
e.Graphics.FillRectangle(Brushes.White, this.ClientRectangle);
}
e.Graphics.DrawRectangle(Pens.Black, new Rectangle(0, 0, this.Width - 1, this.Height - 1));
//绘制按钮的文本
StringFormat stringFormat = new StringFormat();
stringFormat.Alignment = StringAlignment.Center;
stringFormat.LineAlignment = StringAlignment.Center;
e.Graphics.DrawString(this.Text, this.Font, new SolidBrush(this.ForeColor), this.ClientRectangle, stringFormat);
}
//重写基类的 OnMouseEnter 方法,处理鼠标进入控件的事件
protected override void OnMouseEnter(EventArgs e)
{
base.OnMouseEnter(e);
_isMouseOver = true;
this.Invalidate();
}
//重写基类的 OnMouseLeave 方法,处理鼠标离开控件的事件
protected override void OnMouseLeave(EventArgs e)
{
base.OnMouseLeave(e);
_isMouseOver = false;
this.Invalidate();
}
//重写基类的 OnMouseDown 方法,处理鼠标按下控件的事件
protected override void OnMouseDown(MouseEventArgs e)
{
base.OnMouseDown(e);
_isMouseDown = true;
this.Invalidate();
}
//重写基类的 OnMouseUp 方法,处理鼠标松开控件的事件
protected override void OnMouseUp(MouseEventArgs e)
{
base.OnMouseUp(e);
_isMouseDown = false;
this.Invalidate();
}
}
```
在上面的代码中,我们重写了基类的 OnPaint、OnMouseEnter、OnMouseLeave、OnMouseDown 和 OnMouseUp 方法,分别处理了控件的绘制、鼠标进入、鼠标离开、鼠标按下和鼠标松开事件。在 OnPaint 方法中,我们使用 Graphics 对象绘制了控件的背景、边框和文本。
4.将控件添加到工具箱
完成自定义控件的编写后,我们需要将它添加到工具箱中,以便在 WinForm 窗体设计器中使用。
在类库项目中,右键单击“引用”->“添加引用”,然后选择“浏览”,找到刚才创建的类库项目生成的 DLL 文件,添加引用。
接着,在类库项目中,右键单击“ButtonEx.cs”文件,选择“属性”,将“生成操作”设置为“编译”。
然后,打开 WinForm 窗体设计器,右键单击工具箱中的空白区域,选择“选择项”,在“选择工具箱项”对话框中选择“浏览”,找到刚才创建的类库项目生成的 DLL 文件,添加到工具箱中。
这样,我们就可以在 WinForm 窗体设计器中使用自定义的控件了。
阅读全文
相关推荐
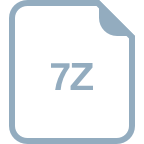
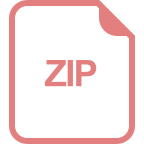
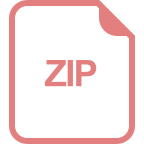
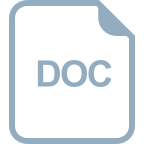
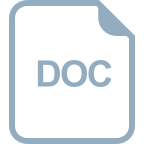
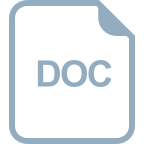
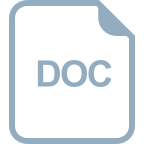
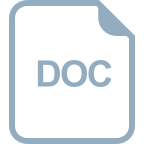





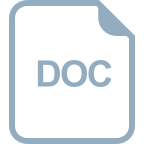