采用promise对象,实现执行一个加法的函数
时间: 2023-05-31 18:05:23 浏览: 23
function add(a, b) {
return new Promise((resolve, reject) => {
if (typeof a !== "number" || typeof b !== "number") {
reject("参数必须为数字");
} else {
resolve(a + b);
}
});
}
// 调用
add(1, 2)
.then((result) => {
console.log(result); // 输出 3
})
.catch((error) => {
console.error(error); // 输出错误信息
});
相关问题
采用promise对象,实现执行一个加法的函数,如果成功运行这个函数,则将执行完后返回加法的结果
function add(a, b) {
return new Promise(function(resolve, reject) {
if (typeof a !== 'number' || typeof b !== 'number') {
reject(new Error('Inputs must be numbers'));
} else {
resolve(a + b);
}
});
}
add(1, 2)
.then(function(result) {
console.log(result); // 3
})
.catch(function(error) {
console.error(error);
});
promise执行加法函数
以下是一个使用Promise执行加法函数的示例:
```javascript
function add(a, b) {
return new Promise((resolve, reject) => {
if (typeof a !== 'number' || typeof b !== 'number') {
reject('Both arguments must be numbers');
} else {
const sum = a + b;
resolve(sum);
}
})
}
add(2, 3)
.then(result => console.log(result)) // Output: 5
.catch(error => console.error(error));
add('2', 3)
.then(result => console.log(result))
.catch(error => console.error(error)); // Output: 'Both arguments must be numbers'
```
在这个示例中,`add`函数返回了一个Promise对象。如果两个参数都是数字,则会计算它们的和并通过`resolve`方法将结果传递给`.then`回调函数。如果其中一个参数不是数字,则会通过`reject`方法将错误消息传递给`.catch`回调函数。在Promise对象被创建后,我们可以使用`.then`和`.catch`方法来处理成功和失败的情况。
阅读全文
相关推荐
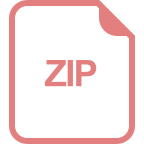
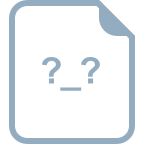

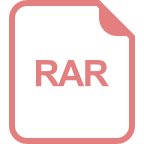
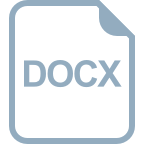
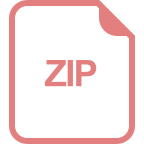
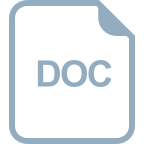
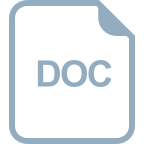
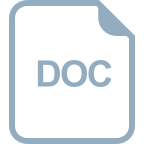
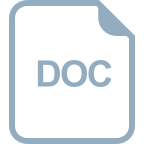
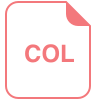
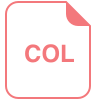
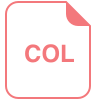
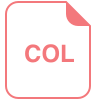
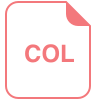
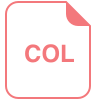