以C结构体或C++的“类”代替“第2章中复杂数据类型”,实现“链式线性表”,编写下面6个接口函数:CreateList、ListPrint、GetElem、ListLength、ListInsert、ListDelete
时间: 2023-05-28 08:07:15 浏览: 32
首先定义链式线性表节点的结构体:
```c
typedef struct ListNode {
int val; // 数据域
struct ListNode* next; // 指针域
} ListNode;
```
接下来,我们定义一个链式线性表的结构体,包含链表头指针和链表长度:
```c
typedef struct {
ListNode* head; // 头指针
int length; // 链表长度
} LinkedList;
```
然后,实现CreateList函数,用于创建一个空的链式线性表:
```c
void CreateList(LinkedList* list) {
list->head = NULL; // 头指针置空
list->length = 0; // 长度为0
}
```
接下来,实现ListPrint函数,用于输出链式线性表的所有元素:
```c
void ListPrint(LinkedList* list) {
ListNode* p = list->head; // 从头节点开始遍历
while (p != NULL) { // 遍历到链表尾节点
printf("%d ", p->val); // 输出当前节点的值
p = p->next; // 移动到下一个节点
}
printf("\n"); // 换行
}
```
然后,实现GetElem函数,用于获取链式线性表中指定位置的元素:
```c
ListNode* GetElem(LinkedList* list, int index) {
if (index < 0 || index >= list->length) { // 判断索引是否越界
return NULL;
}
ListNode* p = list->head; // 从头节点开始遍历
int i = 0;
while (i < index) { // 遍历到指定位置的前一个节点
p = p->next;
i++;
}
return p; // 返回指定位置的节点
}
```
接下来,实现ListLength函数,用于获取链式线性表的长度:
```c
int ListLength(LinkedList* list) {
return list->length; // 直接返回链表长度
}
```
然后,实现ListInsert函数,用于在链式线性表中指定位置插入元素:
```c
void ListInsert(LinkedList* list, int index, int val) {
if (index < 0 || index > list->length) { // 判断索引是否越界
return;
}
ListNode* node = malloc(sizeof(ListNode)); // 新建节点
node->val = val; // 赋值
if (index == 0) { // 插入在头部
node->next = list->head;
list->head = node;
} else { // 插入在中间或尾部
ListNode* p = GetElem(list, index - 1); // 获取插入位置的前一个节点
node->next = p->next;
p->next = node;
}
list->length++; // 长度加1
}
```
最后,实现ListDelete函数,用于删除链式线性表中指定位置的元素:
```c
void ListDelete(LinkedList* list, int index) {
if (index < 0 || index >= list->length) { // 判断索引是否越界
return;
}
if (index == 0) { // 删除头节点
ListNode* p = list->head;
list->head = p->next;
free(p);
} else { // 删除中间或尾节点
ListNode* p = GetElem(list, index - 1); // 获取删除位置的前一个节点
ListNode* q = p->next; // 获取删除位置的节点
p->next = q->next;
free(q);
}
list->length--; // 长度减1
}
```
完整代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val; // 数据域
struct ListNode* next; // 指针域
} ListNode;
typedef struct {
ListNode* head; // 头指针
int length; // 链表长度
} LinkedList;
void CreateList(LinkedList* list) {
list->head = NULL; // 头指针置空
list->length = 0; // 长度为0
}
void ListPrint(LinkedList* list) {
ListNode* p = list->head; // 从头节点开始遍历
while (p != NULL) { // 遍历到链表尾节点
printf("%d ", p->val); // 输出当前节点的值
p = p->next; // 移动到下一个节点
}
printf("\n"); // 换行
}
ListNode* GetElem(LinkedList* list, int index) {
if (index < 0 || index >= list->length) { // 判断索引是否越界
return NULL;
}
ListNode* p = list->head; // 从头节点开始遍历
int i = 0;
while (i < index) { // 遍历到指定位置的前一个节点
p = p->next;
i++;
}
return p; // 返回指定位置的节点
}
int ListLength(LinkedList* list) {
return list->length; // 直接返回链表长度
}
void ListInsert(LinkedList* list, int index, int val) {
if (index < 0 || index > list->length) { // 判断索引是否越界
return;
}
ListNode* node = malloc(sizeof(ListNode)); // 新建节点
node->val = val; // 赋值
if (index == 0) { // 插入在头部
node->next = list->head;
list->head = node;
} else { // 插入在中间或尾部
ListNode* p = GetElem(list, index - 1); // 获取插入位置的前一个节点
node->next = p->next;
p->next = node;
}
list->length++; // 长度加1
}
void ListDelete(LinkedList* list, int index) {
if (index < 0 || index >= list->length) { // 判断索引是否越界
return;
}
if (index == 0) { // 删除头节点
ListNode* p = list->head;
list->head = p->next;
free(p);
} else { // 删除中间或尾节点
ListNode* p = GetElem(list, index - 1); // 获取删除位置的前一个节点
ListNode* q = p->next; // 获取删除位置的节点
p->next = q->next;
free(q);
}
list->length--; // 长度减1
}
int main() {
LinkedList list;
CreateList(&list);
ListInsert(&list, 0, 1);
ListInsert(&list, 0, 2);
ListInsert(&list, 2, 3);
ListPrint(&list); // 输出:2 1 3
ListDelete(&list, 1);
ListPrint(&list); // 输出:2 3
return 0;
}
```
相关推荐
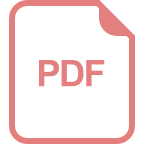
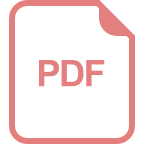
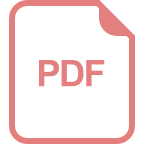


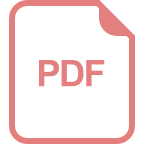
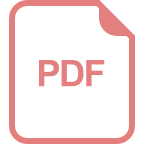
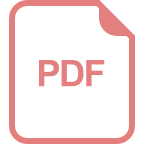
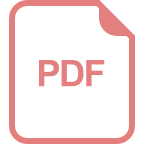
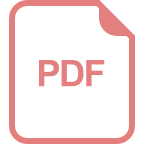
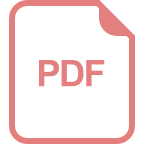
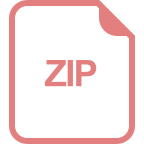
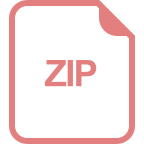