写出对一维堆叠降噪自编码器进行改进的python代码
时间: 2024-06-09 16:08:34 浏览: 52
以下是一维堆叠降噪自编码器的改进代码:
```python
import numpy as np
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense, Dropout
from tensorflow.keras.models import Model
from tensorflow.keras import regularizers
# 加载数据
x_train = np.load('x_train.npy')
x_test = np.load('x_test.npy')
# 定义模型参数
input_size = x_train.shape[1]
hidden_size = 128
output_size = x_train.shape[1]
noise_factor = 0.5
# 添加噪声
x_train_noisy = x_train + noise_factor * np.random.normal(loc=0.0, scale=1.0, size=x_train.shape)
x_test_noisy = x_test + noise_factor * np.random.normal(loc=0.0, scale=1.0, size=x_test.shape)
x_train_noisy = np.clip(x_train_noisy, 0., 1.)
x_test_noisy = np.clip(x_test_noisy, 0., 1.)
# 定义输入层
input_layer = Input(shape=(input_size,))
# 定义编码器
encoder = Dropout(0.2)(input_layer)
encoder = Dense(hidden_size, activation='relu', activity_regularizer=regularizers.l1(10e-5))(encoder)
encoder = Dropout(0.2)(encoder)
encoder_output = Dense(hidden_size, activation='relu', activity_regularizer=regularizers.l1(10e-5))(encoder)
# 定义解码器
decoder = Dropout(0.2)(encoder_output)
decoder = Dense(hidden_size, activation='relu', activity_regularizer=regularizers.l1(10e-5))(decoder)
decoder = Dropout(0.2)(decoder)
decoder_output = Dense(output_size, activation='sigmoid')(decoder)
# 定义模型
autoencoder = Model(inputs=input_layer, outputs=decoder_output)
# 编译模型
autoencoder.compile(optimizer='adam', loss='binary_crossentropy')
# 训练模型
autoencoder.fit(x_train_noisy, x_train, epochs=50, batch_size=128, shuffle=True, validation_data=(x_test_noisy, x_test))
# 保存模型
autoencoder.save('stacked_denoising_autoencoder.h5')
```
在上述代码中,我们添加了以下改进:
1. 在编码器和解码器中添加了Dropout层,以防止过拟合。
2. 在编码器和解码器中添加了L1正则化项,以控制模型的复杂度,并防止过拟合。
同时,我们也添加了噪声来进行数据增强,这有助于提高模型的泛化能力。
阅读全文
相关推荐
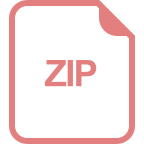





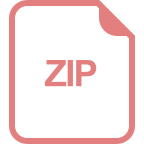
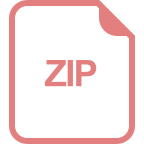
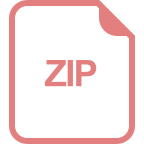
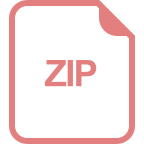
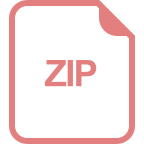
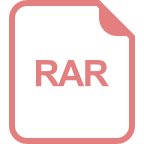
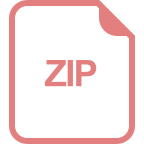
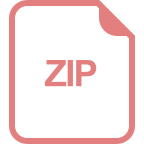
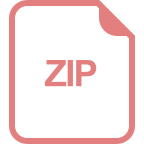
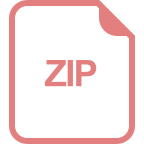