import matplotlib.pyplot as plt import numpy as np from itertools import product from sklearn.neighbors import KNeighborsClassifier n_points = 100 X1 = np.random.multivariate_normal([1,50], [[1,0],[0,10]], n_points) X2 = np.random.multivariate_normal([2,50], [[1,0],[0,10]], n_points) X = np.concatenate([X1,X2]) y = np.array([0]*n_points + [1]*n_points) print (X.shape, y.shape) clfs = [] neighbors = [1,3,5,9,11,13,15,17,19] for i in range(len(neighbors)): clfs.append(KNeighborsClassifier(n_neighbors=neighbors[i]).fit(X,y)) x_min, x_max = X[:, 0].min() - 1, X[:, 0].max() + 1 y_min, y_max = X[:, 1].min() - 1, X[:, 1].max() + 1 xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.1), np.arange(y_min, y_max, 0.1)) f, axarr = plt.subplots(3, 3, sharex='col', sharey='row', figsize=(15, 12)) for idx, clf, tt in zip(product([0, 1, 2], [0, 1, 2]), clfs, ['KNN (k=%d)' % k for k in neighbors]): Z = clf.predict(np.c_[xx.ravel(), yy.ravel()]) Z = Z.reshape(xx.shape) axarr[idx[0], idx[1]].contourf(xx, yy, Z, alpha=0.4) axarr[idx[0], idx[1]].scatter(X[:, 0], X[:, 1], c=y, s=20, edgecolor='k') axarr[idx[0], idx[1]].set_title(tt) plt.show()帮我逐句解析代码
时间: 2023-07-02 09:06:42 浏览: 65
1. 首先导入所需要的包:
```python
import matplotlib.pyplot as plt
import numpy as np
from itertools import product
from sklearn.neighbors import KNeighborsClassifier
```
2. 定义数据集的大小和两个高斯分布:
```python
n_points = 100
X1 = np.random.multivariate_normal([1,50], [[1,0],[0,10]], n_points)
X2 = np.random.multivariate_normal([2,50], [[1,0],[0,10]], n_points)
X = np.concatenate([X1,X2])
y = np.array([0]*n_points + [1]*n_points)
```
这里,我们生成了两个高斯分布,其中一个的均值为 (1, 50),协方差矩阵为 [[1,0],[0,10]],另一个的均值为 (2, 50),协方差矩阵也为 [[1,0],[0,10]]。这两个高斯分布各自生成了100个点,最后将它们拼接在一起形成数据集X,并为每个点赋予标签y。
3. 定义不同k值下的KNN分类器:
```python
clfs = []
neighbors = [1,3,5,9,11,13,15,17,19]
for i in range(len(neighbors)):
clfs.append(KNeighborsClassifier(n_neighbors=neighbors[i]).fit(X,y))
```
这里定义了9个KNN分类器,分别以不同的k值进行训练,并将它们存储在列表clfs中。
4. 定义画图所需的x, y轴范围和网格:
```python
x_min, x_max = X[:, 0].min() - 1, X[:, 0].max() + 1
y_min, y_max = X[:, 1].min() - 1, X[:, 1].max() + 1
xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.1), np.arange(y_min, y_max, 0.1))
```
这里,我们首先获取x, y轴的最小值和最大值,然后在这个范围内生成一个网格,步长为0.1。
5. 定义画图布局:
```python
f, axarr = plt.subplots(3, 3, sharex='col', sharey='row', figsize=(15, 12))
```
这里,我们创建了一个3x3的画布,并将其存储在变量f中,同时也获取了子图axarr。
6. 循环遍历每个KNN分类器,并将其结果可视化:
```python
for idx, clf, tt in zip(product([0, 1, 2], [0, 1, 2]), clfs, ['KNN (k=%d)' % k for k in neighbors]):
Z = clf.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
axarr[idx[0], idx[1]].contourf(xx, yy, Z, alpha=0.4)
axarr[idx[0], idx[1]].scatter(X[:, 0], X[:, 1], c=y, s=20, edgecolor='k')
axarr[idx[0], idx[1]].set_title(tt)
plt.show()
```
这里,我们使用了zip和product函数,将每个分类器、子图、标题对应起来。对于每个分类器,我们首先用np.c_将网格上的点变成一个点集,然后用predict函数进行分类,并将结果reshape成网格的形状。接着,我们使用contourf函数将分类结果可视化,同时用scatter函数将数据集X中的点画出来。最后,我们用set_title函数设置每个子图的标题。最终,我们将所有子图显示出来。
相关推荐
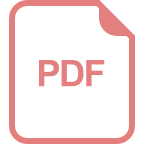
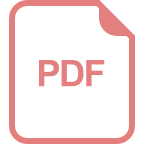
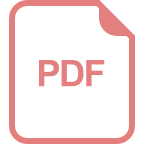















