那如何判断字符串中仅存在注释, 且将注释代码单独取出
时间: 2023-12-13 07:04:03 浏览: 38
如果要判断一个字符串中仅存在注释,可以使用如下代码:
```java
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class CommentChecker {
public static void main(String[] args) {
String str = "// This is a comment";
boolean isCommentOnly = checkIfCommentOnly(str);
System.out.println(isCommentOnly); // 输出 true
}
private static boolean checkIfCommentOnly(String str) {
// 匹配 // 或 /* */ 形式的注释
Pattern pattern = Pattern.compile("(//.*)|(/\\*.*\\*/)");
Matcher matcher = pattern.matcher(str);
return matcher.matches(); // 如果字符串中仅存在注释则返回 true,否则返回 false
}
}
```
在这个例子中,我们使用 `checkIfCommentOnly` 方法来判断字符串中是否仅存在注释。在该方法中,我们使用正则表达式匹配注释,并使用 `Matcher` 对象的 `matches()` 方法来判断字符串中是否仅存在注释。如果字符串中仅存在注释,则返回 `true`,否则返回 `false`。
如果要将注释代码单独取出,可以使用如下代码:
```java
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class CommentChecker {
public static void main(String[] args) {
String str = "// This is a comment";
String comment = extractComment(str);
System.out.println(comment); // 输出 "// This is a comment"
}
private static String extractComment(String str) {
// 匹配 // 或 /* */ 形式的注释
Pattern pattern = Pattern.compile("(//.*)|(/\\*.*\\*/)");
Matcher matcher = pattern.matcher(str);
if (matcher.find()) {
return matcher.group(); // 返回注释内容
} else {
return null; // 如果没有匹配到注释,则返回 null
}
}
}
```
在这个例子中,我们使用 `extractComment` 方法将注释代码从字符串中单独取出。在该方法中,我们使用正则表达式匹配注释,并使用 `Matcher` 对象的 `find()` 方法查找字符串中是否有匹配的注释。如果有匹配的注释,则使用 `group()` 方法返回注释内容,否则返回 `null`。
相关推荐
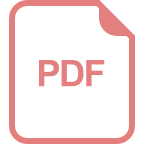
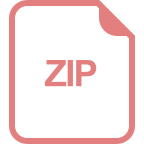














