编写一函数,由实参传来一个字符串,统计此字符串中字母、数字、空格和其它字符的个数,在主函数中输入字符串以及输出上述结果。 只要结果,别输出什么提示信息
时间: 2023-09-10 17:02:13 浏览: 141
### 回答1:
主要思路是用循环遍历输入的字符串,然后根据字符类型累加出现次数,最后输出结果即可。具体实现可以使用字典存储不同种类字符的出现次数,也可以使用列表存储字符类型的枚举值(例如用0表示字母,1表示数字等),再使用列表统计每种类型字符出现次数。无论使用何种方式,都需要注意遍历时需要忽略空格等无效字符。
### 回答2:
可以编写一个函数来统计给定字符串中字母、数字、空格和其他字符的个数,并在主函数中输入字符串,然后输出统计结果。
以下是使用Python编写的相应代码:
```python
def count_characters(string):
letters = 0
digits = 0
spaces = 0
others = 0
for char in string:
if char.isalpha(): # 判断字符是否为字母
letters += 1
elif char.isdigit(): # 判断字符是否为数字
digits += 1
elif char.isspace(): # 判断字符是否为空格
spaces += 1
else:
others += 1
return letters, digits, spaces, others
if __name__ == "__main__":
input_string = input("请输入字符串:")
letter_count, digit_count, space_count, other_count = count_characters(input_string)
print(letter_count, digit_count, space_count, other_count)
```
上述代码中,`count_characters`函数用于接收一个字符串作为参数,统计该字符串中字母、数字、空格和其他字符的个数,并返回结果。在主函数中,用户需要输入一个字符串,然后调用`count_characters`函数并将结果打印出来。
希望这个回答对您有帮助!
### 回答3:
可以编写一个函数来实现统计字符串中字母、数字、空格和其他字符的个数。以下是一个示例代码:
```python
def count_chars(input_str):
letter_count = 0
digit_count = 0
space_count = 0
other_count = 0
for char in input_str:
if char.isalpha():
letter_count += 1
elif char.isdigit():
digit_count += 1
elif char.isspace():
space_count += 1
else:
other_count += 1
return letter_count, digit_count, space_count, other_count
input_str = input("请输入一个字符串:")
result = count_chars(input_str)
print("字母的个数:", result[0])
print("数字的个数:", result[1])
print("空格的个数:", result[2])
print("其他字符的个数:", result[3])
```
使用该函数,我们可以通过主函数输入一个字符串,并输出字母、数字、空格和其他字符的个数。
阅读全文
相关推荐















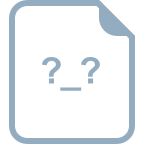