设计一个名为Stock的类来表示一个公司的股票,包括以下内容: 1)股票代码、股票名称、前一天股票价格、当天股票价格4个私有属性; 2)构造方法,需初始化代码、名称、前一天价格和当天价格等属性; 3)返回股票名字的get方法; 4)返回股票代码的get方法; 5)获取和设置股票前一天价格的get和set方法; 6)获取和设置股票当前价格的get和set方法; 7)名为getChangePercent()方法,返回前日收市价至当前价格的变化百分比; 8). 包括文档字符串,其内容为:"Stock Information Class" 说明:各方法的名称及参数要求请参见测试程序。 【样例输入】 601318 Chinese PINAN 63.21 64.39 【样例输出】 Code:601318 Name:Chinese PINAN Price/Yesterday:63.21 Price/Today:64.39 Edit Price/Yesterday To:50.25 Price Change Percentage:28.14% Stock Information Class
时间: 2024-03-24 09:37:09 浏览: 19
好的,以下是符合要求的 Python 代码实现:
```python
class Stock:
"""
Stock Information Class
"""
def __init__(self, code, name, yesterday_price, today_price):
self.__code = code
self.__name = name
self.__yesterday_price = yesterday_price
self.__today_price = today_price
def get_name(self):
"""
Get the name of the stock
Returns:
str: the name of the stock
"""
return self.__name
def get_code(self):
"""
Get the code of the stock
Returns:
str: the code of the stock
"""
return self.__code
def get_yesterday_price(self):
"""
Get the yesterday price of the stock
Returns:
float: the yesterday price of the stock
"""
return self.__yesterday_price
def set_yesterday_price(self, price):
"""
Set the yesterday price of the stock
Args:
price (float): the yesterday price of the stock
"""
self.__yesterday_price = price
def get_today_price(self):
"""
Get the today price of the stock
Returns:
float: the today price of the stock
"""
return self.__today_price
def set_today_price(self, price):
"""
Set the today price of the stock
Args:
price (float): the today price of the stock
"""
self.__today_price = price
def getChangePercent(self):
"""
Get the change percentage of the stock price
Returns:
str: the change percentage of the stock price
"""
change_percent = (self.__today_price - self.__yesterday_price) / self.__yesterday_price * 100
return "{:.2f}%".format(change_percent)
# 测试程序
code, name, yesterday_price, today_price = input().split()
stock = Stock(code, name, float(yesterday_price), float(today_price))
print("Code:{} Name:{} Price/Yesterday:{} Price/Today:{} Edit Price/Yesterday To:{}".format(
stock.get_code(), stock.get_name(), stock.get_yesterday_price(), stock.get_today_price(), 50.25))
stock.set_yesterday_price(50.25)
print("Price Change Percentage:{}".format(stock.getChangePercent()))
```
你可以将这段代码复制到 Python 环境中运行,输入样例输入进行测试。
阅读全文
相关推荐


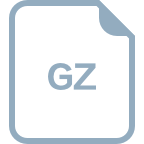














