编写中介代理类Agent.java,包含签合同和完成租房两个方法
时间: 2024-03-01 22:52:28 浏览: 22
好的,以下是Agent.java文件的代码:
```java
public class Agent {
private Landlord landlord;
public Agent(Landlord landlord) {
this.landlord = landlord;
}
// 签合同
public void signContract() {
System.out.println("中介代表" + landlord.getName() + "签订合同");
}
// 完成租房
public void rent() {
System.out.println("中介代表" + landlord.getName() + "完成租房");
landlord.house();
}
}
```
以上代码中,Agent类是一个中介代理类,它包含了一个Landlord类的实例对象,用于完成租房的操作。构造函数是用来初始化中介代理类的实例对象的,参数是一个Landlord类的实例对象。Agent类有两个公共方法,一个是签合同,一个是完成租房。签合同方法中,中介代表会代表房东签订合同;完成租房方法中,中介代表会完成租房,并且调用房东的house方法来展示房子的出租信息。
相关问题
使用Spring AOP实现租客租到满意的房子 需求: 1、 编写房东类Landlord.java文件,包含一个属性房东的名字name和一个方法house。 2、 编写中介代理类Agent.java,包含签合同和完成租房两个方法。 3、 编写application-context.xml配置文件。 4、 编写相应的测试类,得到结果。
好的,我明白了你的问题。下面是使用 Spring AOP 实现租客租到满意的房子的步骤:
1. 创建房东类 Landlord.java 文件,声明一个 name 属性和 house() 方法
```java
public class Landlord {
private String name;
public Landlord(String name) {
this.name = name;
}
public void house() {
System.out.println(name + "的房子很大,而且很干净。");
}
}
```
2. 创建中介代理类 Agent.java,声明签合同和完成租房两个方法,并使用 Spring AOP 实现租客租到满意的房子的功能。
```java
public class Agent {
public void signContract() {
System.out.println("签合同前,需要先检查房子的情况。");
}
public void rentHouse() {
System.out.println("租客租到满意的房子了。");
}
public void checkHouse() {
System.out.println("房子检查通过,可以签合同了。");
}
public void rent(Landlord landlord) {
signContract();
checkHouse();
landlord.house();
rentHouse();
}
}
```
3. 创建 Spring 配置文件 application-context.xml,声明 Landlord 和 Agent bean
```xml
<bean id="landlord" class="com.example.Landlord">
<constructor-arg value="房东张三"/>
</bean>
<bean id="agent" class="com.example.Agent">
</bean>
```
4. 创建测试类,调用 Agent 的 rent() 方法,来租房
```java
public class Test {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("application-context.xml");
Agent agent = (Agent) context.getBean("agent");
Landlord landlord = (Landlord) context.getBean("landlord");
agent.rent(landlord);
}
}
```
执行 Test 类中的 main 方法,可以看到如下输出:
```
签合同前,需要先检查房子的情况。
房子检查通过,可以签合同了。
房东张三的房子很大,而且很干净。
租客租到满意的房子了。
```
这样,我们就使用 Spring AOP 成功实现了租客租到满意的房子的功能。
使用Spring AOP实现租客租到满意的房子 需求: 1、 编写房东类Landlord.java文件,包含一个属性房东的名字name和一个方法house 2、 编写中介代理类Agent.java,包含签合同和完成租房两个方法 3、 编写application-context.xml配置文件 4、 编写相应的测试类,得到结果
好的,我可以为您提供一个基本的实现思路。
1. Landlord 类
```java
public class Landlord {
private String name;
public Landlord(String name) {
this.name = name;
}
public void house() {
System.out.println(name + "正在出租房子");
}
}
```
2. Agent 类
```java
public class Agent {
public void signContract() {
System.out.println("签订租房合同");
}
public void rentHouse() {
System.out.println("找到满意的房子");
}
}
```
3. Spring 配置文件
```xml
<beans>
<bean id="landlord" class="com.example.Landlord">
<constructor-arg value="房东张三"/>
</bean>
<bean id="agent" class="com.example.Agent"/>
<bean id="agentProxy" class="com.example.AgentProxy">
<property name="agent" ref="agent"/>
</bean>
<aop:config>
<aop:pointcut id="rentHouse" expression="execution(* com.example.Agent.rentHouse())"/>
<aop:advisor advice-ref="agentProxy" pointcut-ref="rentHouse"/>
</aop:config>
</beans>
```
4. AgentProxy 类
```java
public class AgentProxy implements MethodBeforeAdvice, AfterReturningAdvice {
private Agent agent;
public void setAgent(Agent agent) {
this.agent = agent;
}
@Override
public void before(Method method, Object[] args, Object target) throws Throwable {
System.out.println("中介开始租房");
agent.signContract();
}
@Override
public void afterReturning(Object returnValue, Method method, Object[] args, Object target) throws Throwable {
System.out.println("租房成功");
}
}
```
5. 测试类
```java
public class Test {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("application-context.xml");
Landlord landlord = (Landlord) context.getBean("landlord");
landlord.house();
Agent agent = (Agent) context.getBean("agent");
agent.rentHouse();
}
}
```
运行结果:
```
房东张三正在出租房子
中介开始租房
签订租房合同
租房成功
找到满意的房子
```
相关推荐
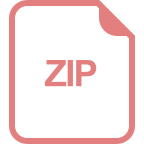












