.net 6 jsonarray 追加 jsonnode
时间: 2023-10-23 07:08:44 浏览: 164
可以通过以下代码在JsonArray中追加一个JsonNode节点:
```
JsonNode node = ... ; // 待追加的节点
JsonArray array = ... ; // 目标数组
array.add(node);
```
需要引入以下命名空间:
```
using System.Text.Json;
using System.Text.Json.Serialization;
```
然后就可以使用以上代码进行追加操作了。
相关问题
.net 解析JSONArray
在.NET中,如果你想要解析JSON数组,可以使用Json.NET库(也称为Newtonsoft.Json),这是一个流行的第三方JSON处理框架。以下是一个简单的步骤:
1. 首先,你需要安装Json.NET。如果你使用的是NuGet包管理器,可以在项目中添加`Newtonsoft.Json`依赖项。
```csharp
Install-Package Newtonsoft.Json
```
2. 然后,在C#代码中,你可以使用`JArray`类来读取和操作JSON数组。假设你有一个包含JSON数据的字符串`jsonString`:
```csharp
using Newtonsoft.Json.Linq;
// JSON字符串示例
string jsonString = @"[{'name': 'John', 'age': 30}, {'name': 'Jane', 'age': 25}]";
// 解析JSON字符串为JArray
JArray jsonArray = JArray.Parse(jsonString);
// 访问和操作数组元素
foreach (JObject jsonObject in jsonArray)
{
string name = jsonObject["name"].ToString();
int age = jsonObject["age"].ToObject<int>();
Console.WriteLine($"Name: {name}, Age: {age}");
}
// 提供更多的操作,如查找、过滤等
JToken searchResult = jsonArray.FirstOrDefault(j => j["name"] == "John");
if (searchResult != null)
{
// 处理找到的结果
}
```
.Net JsonArray
JsonArray is a class in the .NET framework that is used to represent a collection of JSON objects or values. It is part of the Newtonsoft.Json namespace and can be used to parse, create, and manipulate JSON data.
The JsonArray class provides methods to add, remove, and access elements of the array. It also supports iteration through the elements using a foreach loop. The class can be used to serialize JSON data to a string or deserialize JSON data from a string.
Here is an example of creating a JsonArray object:
```
using Newtonsoft.Json.Linq;
JArray jsonArray = new JArray();
jsonArray.Add("item1");
jsonArray.Add("item2");
jsonArray.Add("item3");
```
In this example, we create a new JsonArray object and add three string values to it. The resulting JsonArray object would look like this:
```
["item1", "item2", "item3"]
```
Overall, the JsonArray class is a useful tool for working with JSON data in .NET applications.
阅读全文
相关推荐
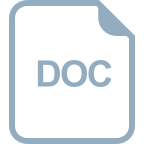
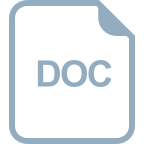
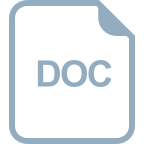
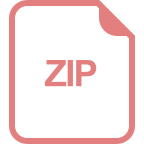












