java实现将base64转为文件并下载到本地电脑D盘指定文件夹下
时间: 2024-09-15 16:04:10 浏览: 45
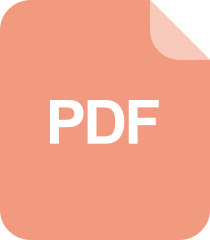
java将图片转为base64返回给前端

在Java中,你可以使用`java.util.Base64`库进行Base64编码,然后利用`java.io.FileOutputStream`和`java.net.URLConnection`来下载文件。以下是基本的步骤:
首先,你需要对Base64字符串解码得到原始数据,然后创建一个新的FileOutputStream并将其指向目标文件路径,最后从URL读取内容并写入文件。
```java
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.net.URL;
public class Base64ToFile {
public static void downloadAndDecode(String base64Data, String targetFolderPath, String fileName) throws IOException {
// 解码Base64字符串
byte[] decodedBytes = Base64.getDecoder().decode(base64Data);
// 创建目标文件路径,如果不存在则先创建
File targetDir = new File(targetFolderPath);
if (!targetDir.exists()) {
targetDir.mkdirs();
}
// 指定完整的文件路径
File outputFile = new File(targetDir, fileName);
try (BufferedInputStream in = new BufferedInputStream(new ByteArrayInputStream(decodedBytes));
FileOutputStream out = new FileOutputStream(outputFile)) {
// 逐字节读取并写入文件
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
System.out.println("File downloaded and decoded successfully.");
}
}
public static void main(String[] args) {
String base64Data = "..."; // 假设这是你要下载的Base64数据
String targetFolderPath = "D:/my_downloads";
String fileName = "example_file.txt";
try {
downloadAndDecode(base64Data, targetFolderPath, fileName);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
记得替换`base64Data`为你实际的Base64编码数据,`targetFolderPath`为你的D盘指定文件夹路径,以及`fileName`为你想要的文件名。
阅读全文
相关推荐
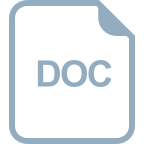
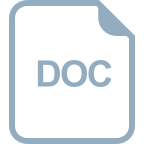
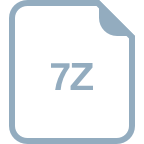
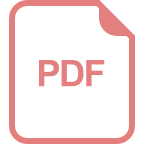
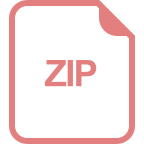
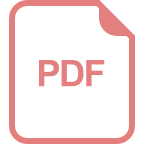
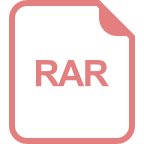
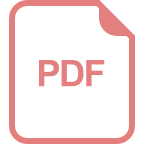
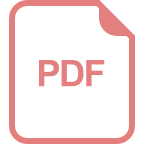
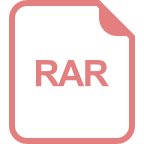
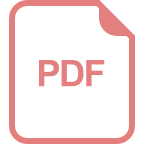
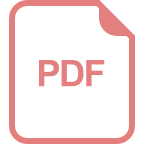
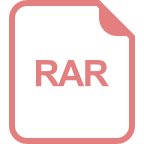
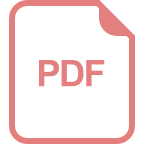
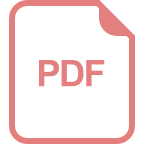
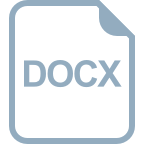
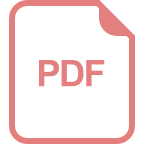