根据后端接口实现vue3增删改查
时间: 2024-12-24 07:23:42 浏览: 2
在Vue3中,实现后端接口的增删改查操作通常需要配合Vuex(状态管理库)和axios(HTTP请求库)。以下是基本步骤:
1. **安装依赖**:
- 安装Vuex: `npm install vuex`
- 如果还没安装axios,可以添加`npm install axios`
2. **创建Vuex store**:
- 创建一个store文件(如store.js),并导入axios实例,初始化state用于存储数据,以及action和mutation来处理API请求。
```javascript
import axios from 'axios';
export const state = {
items: []
};
export const mutations = {
SET_ITEMS(state, response) {
state.items = response.data;
}
};
export const actions = {
async fetchData({ commit }) {
try {
const response = await axios.get('/api/items');
commit('SET_ITEMS', response);
} catch (error) {
console.error('Error fetching data:', error);
}
},
// ...添加其他action(例如addItem、updateItem、deleteItem)
};
```
3. **组件中调用API**:
- 在Vue组件里通过`useStore`注入store,并在生命周期钩子(如`created()`或`mounted()`)里调用actions。
```javascript
import { useStore } from '@/store';
setup() {
const store = useStore();
onMounted(() => {
store.dispatch('fetchData');
});
},
methods: {
addItem(itemData) {
store.dispatch('addItem', itemData);
},
updateItem(id, updatedData) {
store.dispatch('updateItem', { id, data: updatedData });
},
deleteItem(id) {
store.dispatch('deleteItem', id);
}
}
```
4. **响应式更新**:
- 当从后端接口获取的数据变化时,由于Vuex的状态是响应式的,视图会自动更新。
记得根据实际需求替换上述代码中的URL和API路径,同时处理好错误处理和分页、排序等额外功能。
阅读全文
相关推荐
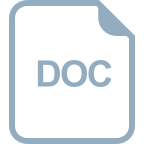
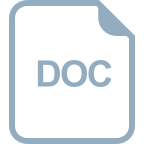
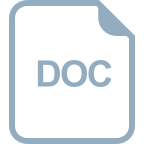

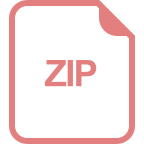
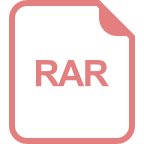
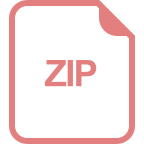
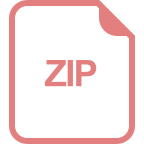
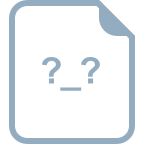
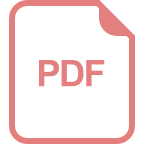
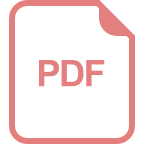
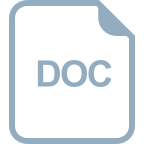






