python fastapi 接口权限
时间: 2024-05-21 12:09:25 浏览: 21
Python FastAPI是一个高性能的Web框架,可以帮助您快速构建REST API。在FastAPI中,您可以使用多种方法来实现API的权限控制,其中一种常用的方法是使用OAuth2认证和JSON Web Token(JWT)。
OAuth2认证是一种流行的开放标准,用于授权,它可以允许第三方应用程序访问受保护的资源。使用OAuth2,您可以为您的API定义各种授权模式,包括密码模式、客户端模式、授权码模式等。通过OAuth2认证,您可以限制哪些用户或者客户端可以访问API的某些部分。
JSON Web Token(JWT)是一种开放标准(RFC 7519),它定义了一种紧凑且自包含的方式来在网络上安全地传输信息。JWT通常用于在不同系统之间共享身份验证信息。在FastAPI中,您可以使用JWT来实现API的权限控制,只有持有有效JWT令牌的用户才能访问受保护的API端点。
除了OAuth2和JWT之外,FastAPI还提供了其他几种权限控制方式,例如HTTP Basic Authentication、HTTP Digest Authentication等。
如果您想要详细了解FastAPI中的权限控制,请参阅FastAPI文档中的相关章节:https://fastapi.tiangolo.com/tutorial/security/
相关问题
python fastapi接口鉴权方法
FastAPI支持基于Bearer Token的鉴权方法,可以使用FastAPI自带的Depends函数结合PyJWT库实现。
以下是一个示例代码:
```python
from fastapi import Depends, FastAPI, HTTPException, status
from fastapi.security import OAuth2PasswordBearer
import jwt
app = FastAPI()
oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token")
def verify_token(token: str):
try:
payload = jwt.decode(token, "secret_key", algorithms=["HS256"])
username = payload.get("sub")
if username is None:
raise HTTPException(
status_code=status.HTTP_401_UNAUTHORIZED,
detail="Invalid authentication credentials",
headers={"WWW-Authenticate": "Bearer"},
)
except jwt.exceptions.DecodeError:
raise HTTPException(
status_code=status.HTTP_401_UNAUTHORIZED,
detail="Invalid authentication credentials",
headers={"WWW-Authenticate": "Bearer"},
)
def get_current_user(token: str = Depends(oauth2_scheme)):
verify_token(token)
return token
@app.get("/protected")
async def protected_route(token: str = Depends(oauth2_scheme)):
return {"token": token}
```
在这个示例中,verify_token函数用于验证JWT Token的有效性,get_current_user函数用于获取当前用户信息,protected_route函数需要鉴权才能访问。在访问protected_route时,会自动调用get_current_user函数进行鉴权。如果鉴权失败,会返回401 Unauthorized错误。
python fastapi 权限控制
Python FastAPI 是一个高性能的 Web 框架,它内置了一套基于 OAuth2 的安全认证和授权机制,可以很方便地实现 API 权限控制。具体来说,FastAPI 基于 OAuth2 规范实现了以下几种授权模式:
1. Password(用户名密码授权):用户提供用户名和密码进行认证,如果认证通过,将返回访问令牌(access token)。
2. Implicit(简化模式):适用于没有后端服务的应用,将直接从前端获取令牌。
3. Authorization Code(授权码模式):适用于需要后端服务的应用,用户在前端应用中获取授权码,然后将授权码交给后端服务获取访问令牌。
4. Client Credentials(客户端授权模式):适用于客户端需要访问受保护的 API 资源的情况,该模式不需要用户参与,直接由客户端向认证服务器请求令牌。
对于每个 API,FastAPI 可以使用 @Depends 装饰器来声明所需的访问令牌类型(比如 OAuth2PasswordBearer),从而实现权限控制。如果请求中未包含正确的访问令牌或者令牌无效,则 FastAPI 将自动返回 401 Unauthorized 或 403 Forbidden 错误。
另外,FastAPI 还支持 JWT(JSON Web Tokens)和 OAuth2 refresh tokens 等高级特性,可以满足各种复杂的权限控制需求。
相关推荐
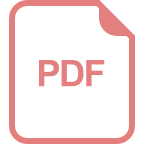
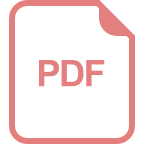
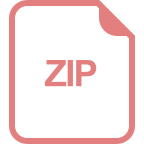
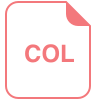
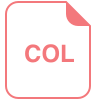
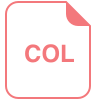
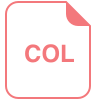
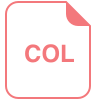







