python HMAC-SM3
时间: 2023-11-04 07:58:28 浏览: 164
Python中的HMAC-SM3是一种基于哈希函数SM3的消息认证码(HMAC)算法。HMAC-SM3可以用于对消息进行完整性验证和身份认证。
要使用HMAC-SM3算法,你可以使用Python的hmac和sm3模块。首先,导入这两个模块:
```python
import hmac
from sm3 import sm3_hash
```
然后,使用hmac.new()函数创建一个HMAC对象,并指定密钥和消息作为参数。密钥和消息都应该是字节类型(bytes)的数据。
```python
key = b'secret_key'
message = b'hello world'
hmac_obj = hmac.new(key, message, digestmod=sm3_hash)
```
最后,使用hexdigest()方法获取HMAC-SM3的摘要值。
```python
digest = hmac_obj.hexdigest()
print(digest)
```
相关问题
js hmac-sm3
以下是使用js实现hmac-sm3的示例代码:
```javascript
const hmac = require('crypto-js/hmac-sm3');
const message = 'hello world';
const key = 'secret key';
const hash = hmac(message, key);
console.log(hash.toString());
```
在上面的代码中,我们使用了`crypto-js`库来实现hmac-sm3算法。首先,我们引入了`hmac-sm3`模块,然后定义了要加密的消息和密钥。接着,我们调用`hmac`函数并传入消息和密钥,该函数将返回一个`WordArray`对象,我们可以通过调用`toString`方法将其转换为字符串并输出。
hmac-sm3代码实现
HMAC-SM3是一种基于SM3 Hash算法的消息认证码。实现HMAC-SM3需要以下步骤:
1. 准备输入数据和HMAC密钥。
2. 对密钥进行预处理,如果密钥长度超过64字节,直接对密钥进行SM3散列;如果密钥长度小于等于64字节,将其追加0x00字节填充至64字节并保存为MK,将0x5C字节追加至MK的每个字节的右侧,并保存为Ko。
3. 将0x36字节追加至MK的每个字节的右侧,并保存为Ki。
4. 将步骤2和步骤3得到的Ko和Ki分别作为秘钥,对输入数据进行SM3散列运算。得到两个SM3散列值H1和H2。
5. 将步骤4得到的H1和H2连接起来作为新的输入数据,再次进行SM3散列运算。得到最终的MAC值。
以下是HMAC-SM3代码实现的示例:
```python
import hashlib
def hmac_sm3(key, message):
key = bytearray.fromhex(key) # 将密钥从十六进制字符串转换为字节数组
if len(key) > 64:
key = hashlib.sm3(key).digest() # 如果密钥长度超过64字节,直接对密钥进行SM3散列
elif len(key) < 64:
key += bytearray(64 - len(key)) # 如果密钥长度小于等于64字节,填充0x00字节至64字节
ko = bytearray(hex(0x5C ^ b)[2:].zfill(2) for b in key) # 生成Ko
ki = bytearray(hex(0x36 ^ b)[2:].zfill(2) for b in key) # 生成Ki
h1 = hashlib.sm3(bytes(ki + message)).digest() # 使用Ki对消息进行SM3散列
h2 = hashlib.sm3(bytes(ko + message)).digest() # 使用Ko对消息进行SM3散列
mac = hashlib.sm3(bytes(h1 + h2)).hexdigest() # 将H1和H2连接后再进行SM3散列,得到最终的MAC值
return mac
key = "0123456789ABCDEF" # HMAC密钥,长度为16个字节
message = "Hello HMAC-SM3!" # 输入数据
mac = hmac_sm3(key, message)
print(mac)
```
此代码示例使用Python编程语言实现HMAC-SM3算法。注意,SM3算法的实现需要依赖相应的密码学库或算法实现。
相关推荐
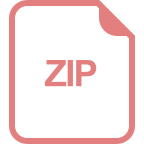
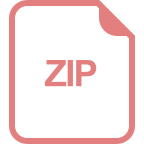












