springboot3.1.1导入依赖
时间: 2023-09-29 16:11:07 浏览: 256
要导入Spring Boot 3.1.1的依赖,你需要在你的项目的pom.xml文件中添加相应的依赖项。以下是一个示例的pom.xml文件,其中包含了Spring Boot 3.1.1的核心依赖项:
```xml
<dependencies>
<!-- Spring Boot 核心依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>3.1.1</version>
</dependency>
<!-- 其他依赖项 -->
<!-- 这里可以添加其他你需要的依赖项 -->
</dependencies>
```
请确保在pom.xml文件中的`<dependencies>`标签内添加上述代码片段,并执行构建操作以下载和安装所需的依赖项。这样你的项目就可以使用Spring Boot 3.1.1了。记得根据你的实际需求,可能需要添加其他依赖项。
相关问题
SpringBoot+Mybatis-plus整合easyExcel批量导入Excel到数据库
好的,这是一个比较常见的需求,我可以给你提供一个简单的实现思路。
1. 首先,我们需要在项目中引入 easyExcel 和 mybatis-plus 的依赖。你可以在 pom.xml 文件中添加以下依赖:
```xml
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>easyexcel</artifactId>
<version>2.1.6</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.1.1</version>
</dependency>
```
2. 然后,我们需要定义一个实体类,用于映射 Excel 中的数据。假设我们要导入的是学生信息,我们可以定义一个 Student 类,如下所示:
```java
@Data
public class Student {
@ExcelProperty("姓名")
private String name;
@ExcelProperty("年龄")
private Integer age;
@ExcelProperty("性别")
private String gender;
@ExcelProperty("班级")
private String className;
}
```
3. 接下来,我们需要定义一个 Controller,用于接收上传的 Excel 文件,并将数据批量插入到数据库中。代码如下:
```java
@RestController
@RequestMapping("/import")
public class ImportController {
@Autowired
private StudentService studentService;
@PostMapping("/excel")
public String importExcel(MultipartFile file) throws Exception {
EasyExcel.read(file.getInputStream(), Student.class, new StudentListener(studentService)).sheet().doRead();
return "导入成功";
}
}
```
4. 在上面的代码中,我们使用了 EasyExcel 提供的 read 方法,将 Excel 文件转换为 Student 类型的数据。同时,我们还定义了一个 StudentListener 类,用于处理读取到的每一行数据。代码如下:
```java
public class StudentListener extends AnalysisEventListener<Student> {
private List<Student> list = new ArrayList<>();
private StudentService studentService;
public StudentListener(StudentService studentService) {
this.studentService = studentService;
}
@Override
public void invoke(Student student, AnalysisContext analysisContext) {
list.add(student);
}
@Override
public void doAfterAllAnalysed(AnalysisContext analysisContext) {
studentService.saveBatch(list);
}
}
```
5. 最后,我们需要在 StudentService 类中添加一个 saveBatch 方法,用于批量插入数据。代码如下:
```java
@Service
public class StudentService extends ServiceImpl<StudentMapper, Student> {
public void saveBatch(List<Student> students) {
saveBatch(students, 100);
}
}
```
这样,我们就完成了使用 SpringBoot+Mybatis-plus+easyExcel 实现批量导入 Excel 到数据库的功能。当然,还有很多细节需要处理,比如 Excel 中的日期等格式问题,你可以根据实际需求进行适当的修改和优化。
阅读全文
相关推荐
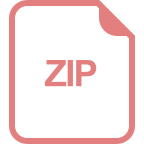
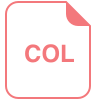
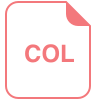
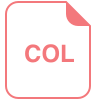
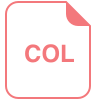
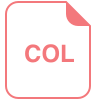
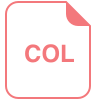
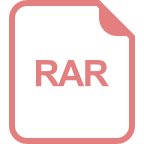
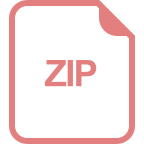
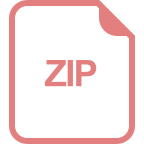
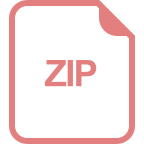