排班系统c语言程序设计
时间: 2023-12-12 16:00:49 浏览: 111
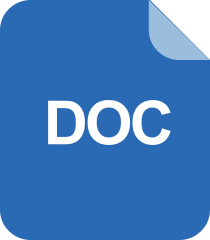
排班系统C语言课程设计.doc

排班系统是一个方便管理和安排员工工作时间的工具。它能够自动分配工作时间和休息时间,并确保所有员工都有合理且公平的工作机会。以下是一个用C语言编写的排班系统的设计示例:
1. 首先,我们需要定义一个员工结构体。该结构体可以包含员工的姓名、工作时间表和休息时间表等信息。
```c
typedef struct {
char name[50];
int work_schedule[7]; // 存储每天的工作时间
int rest_schedule[7]; // 存储每天的休息时间
} Employee;
```
2. 接下来,我们需要编写函数来添加新员工和编辑现有员工的工作和休息时间。我们可以使用数组来存储所有员工的信息。
```c
Employee employees[100]; // 存储所有员工信息的数组
int employee_count = 0; // 记录员工数量
void addEmployee(Employee emp) {
employees[employee_count++] = emp;
}
void editEmployeeSchedule(int empIndex, int dayOfWeek, int workTime, int restTime) {
employees[empIndex].work_schedule[dayOfWeek] = workTime;
employees[empIndex].rest_schedule[dayOfWeek] = restTime;
}
```
3. 然后,我们可以编写函数来计算每位员工的总工作时间和休息时间。
```c
int getTotalWorkTime(Employee emp) {
int total = 0;
for (int i = 0; i < 7; i++) {
total += emp.work_schedule[i];
}
return total;
}
int getTotalRestTime(Employee emp) {
int total = 0;
for (int i = 0; i < 7; i++) {
total += emp.rest_schedule[i];
}
return total;
}
```
4. 最后,我们可以实现一个函数来输出所有员工的工作时间和休息时间。
```c
void printEmployeeSchedules() {
for (int i = 0; i < employee_count; i++) {
printf("员工姓名:%s\n", employees[i].name);
printf("工作时间:");
for (int j = 0; j < 7; j++) {
printf("%d ", employees[i].work_schedule[j]);
}
printf("\n休息时间:");
for (int j = 0; j < 7; j++) {
printf("%d ", employees[i].rest_schedule[j]);
}
printf("\n\n");
}
}
```
以上是一个简单的排班系统的设计示例。通过这个系统,我们可以方便地管理和安排员工的工作时间,确保每位员工都有合理的工作和休息安排。
阅读全文
相关推荐
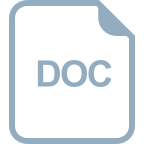
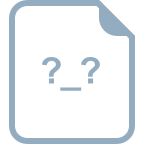







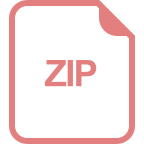
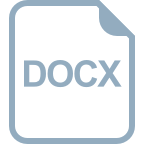
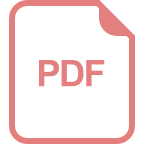
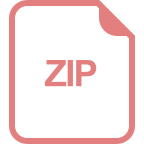
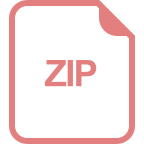
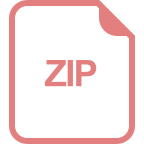
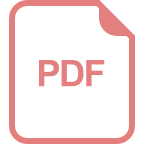
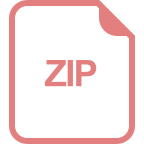