请用C语言编写以下代码:基于字符串模式匹配算法的病毒感染检测问题。要求代码尽量详细
时间: 2024-11-28 19:34:41 浏览: 53
在C语言中,我们可以使用KMP(Knuth-Morris-Pratt)算法或Boyer-Moore算法来进行字符串模式匹配,这两种算法都是为了提高搜索效率。这里我将演示如何使用KMP算法来检测病毒(假设病毒模式是一个固定的字符串):
```c
#include <stdio.h>
#include <string.h>
// 计算部分匹配表
void computeLPS(char pattern[], int lps[]) {
int len = 0, i = 1;
// 初始化前缀和后缀长度数组
lps[0] = 0;
while (i < strlen(pattern)) {
if (pattern[i] == pattern[len]) {
len++;
lps[i] = len;
i++;
} else {
if (len != 0) {
len = lps[len - 1];
} else {
lps[i] = 0;
i++;
}
}
}
}
// KMP字符串匹配函数
int kmpMatch(char text[], char pattern[]) {
int m = strlen(text);
int n = strlen(pattern);
int lps[n], j = 0;
computeLPS(pattern, lps);
for (int i = 0; i < m; i++) {
while (j > 0 && pattern[j] != text[i]) {
j = lps[j - 1];
}
if (pattern[j] == text[i]) {
j++;
}
if (j == n) { // 如果找到了整个模式
printf("Found virus at index %d\n", i - n + 1); // 提供匹配位置
j = lps[j - 1]; // 从失败的位置继续搜索
}
}
return j == 0; // 返回是否找到完整匹配
}
int main() {
char virus[] = "virus_pattern"; // 病毒模式字符串
char file_content[] = "This is a test file with a possible virus."; // 文件内容字符串
if (kmpMatch(file_content, virus)) {
printf("The file contains the virus.\n");
} else {
printf("The file does not contain the virus.\n");
}
return 0;
}
阅读全文
相关推荐











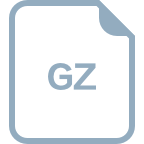


