java中60000条数据怎么快速插入数据库
时间: 2024-09-13 12:16:12 浏览: 47
在Java中向数据库快速插入大量数据,通常可以采取以下几个策略:
1. **批处理插入**:大多数数据库支持批处理操作,可以一次性将多条记录作为一个批次进行插入,这样可以显著减少数据库交互的次数。例如,在JDBC中,可以使用`PreparedStatement`对象的`addBatch()`方法和`executeBatch()`方法来实现批量插入。
2. **关闭自动提交**:在插入数据之前关闭数据库的自动提交模式(`connection.setAutoCommit(false)`),这样可以将多个插入操作合并为一个事务,减少事务的开销,并在全部插入完成后一次性提交。
3. **索引优化**:如果表中有索引,插入数据的速度会减慢。如果允许,在插入数据前可以暂时移除或禁用索引,并在数据全部插入后再重建索引。
4. **调整数据库配置**:根据数据库的配置参数,适当调整缓存大小和连接池设置,以优化大量数据插入的性能。
5. **使用存储过程**:对于一些数据库系统,使用存储过程可能比单条SQL语句执行得更快,可以将数据批量处理后一次性执行。
6. **多线程/多进程插入**:如果硬件资源允许,可以使用多线程或分布式任务将数据分片,利用多个线程或进程并行地向数据库插入数据。
下面是一个简单的Java代码示例,展示如何使用JDBC的批处理插入技术:
```java
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = ...; // 获取数据库连接
connection.setAutoCommit(false); // 关闭自动提交
preparedStatement = connection.prepareStatement("INSERT INTO table_name (column1, column2) VALUES (?, ?)");
for (int i = 0; i < 60000; i++) {
preparedStatement.setString(1, "value1");
preparedStatement.setString(2, "value2");
preparedStatement.addBatch(); // 添加到批处理队列
if (i % 1000 == 0) { // 每1000条执行一次批量插入
preparedStatement.executeBatch(); // 执行批量插入
connection.commit(); // 提交事务
}
}
preparedStatement.executeBatch(); // 执行最后一批数据的插入
connection.commit(); // 提交事务
} catch (Exception e) {
// 处理异常
e.printStackTrace();
} finally {
// 关闭资源
try {
if (preparedStatement != null) preparedStatement.close();
if (connection != null) connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
阅读全文
相关推荐
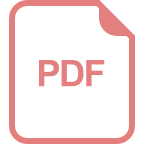
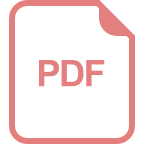
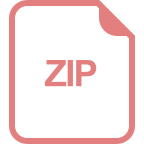
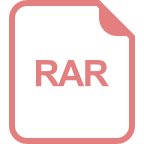
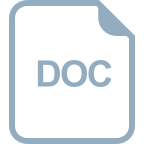
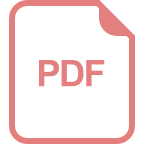
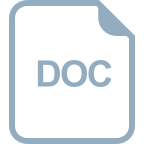
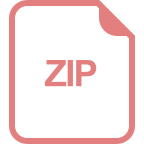
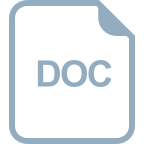
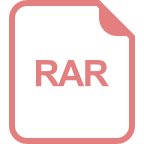
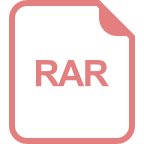
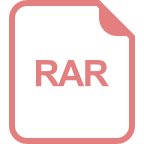
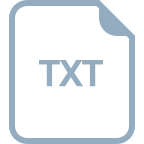
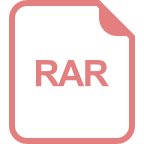
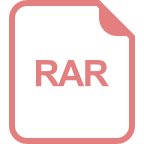
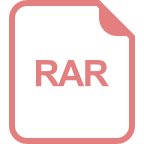
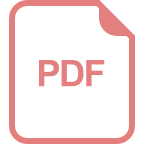