element ui如何关闭某个notification
时间: 2023-11-07 22:28:28 浏览: 138
可以使用 notification 实例的 close 方法来关闭某个 notification,例如:
this.$notify({
title: '提示',
message: '这是一条提示消息',
duration: 0 // duration 设置为 0,表示这个 notification 不会自动关闭
}).$on('close', () => { // 监听 close 事件
console.log('notification 被关闭了')
}).close() // 直接调用 close 方法,手动关闭这个 notification
相关问题
element ui 点赞功能
在 Element UI 中实现点赞功能,可以通过以下步骤进行:
1. 创建一个按钮或图标作为点赞按钮,例如使用 Element UI 提供的 Button 或 Icon 组件。
2. 绑定一个点击事件,当用户点击点赞按钮时触发。
3. 在点击事件处理函数中,根据用户点击状态进行切换。
- 如果用户已经点赞,则取消点赞,删除点赞记录。
- 如果用户还未点赞,则添加点赞记录。
4. 可以使用 Element UI 提供的 Message 或 Notification 组件,在点赞成功或取消点赞成功时进行提示。
下面是一个示例代码:
```html
<template>
<div>
<button @click="toggleLike">
<icon :name="liked ? 'thumbs-up' : 'thumbs-o-up'"></icon>
{{ liked ? '取消点赞' : '点赞' }}
</button>
</div>
</template>
<script>
export default {
data() {
return {
liked: false, // 初始状态为未点赞
};
},
methods: {
toggleLike() {
// 根据当前状态切换点赞状态
this.liked = !this.liked;
// 发送请求到后端保存点赞记录或删除点赞记录
// 在点赞成功或取消点赞成功时进行提示
this.$message.success(this.liked ? '点赞成功' : '取消点赞成功');
},
},
};
</script>
```
请注意,以上代码仅为示例,具体的实现方式可能因项目需求而有所不同。你需要根据自己的项目结构和需求进行相应的调整。
element Notification
您好!对于"element Notification",我可以为您提供一些解释和帮助。Element Notification是Element UI框架中的一个组件,用于展示通知消息。它可以通过弹出框或者顶部横幅的形式向用户显示重要的提示、警告或成功信息。您可以使用Element UI框架提供的API来自定义通知的内容、样式和行为。需要注意的是,具体的使用方法可能会因您的开发环境和技术栈而有所不同。如果您有关于Element Notification的具体问题或者需要更详细的指导,请告诉我。
相关推荐
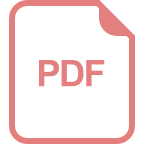
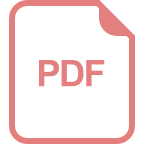
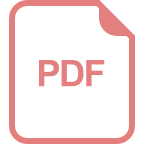
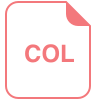
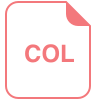
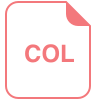
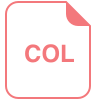
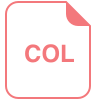







