利用DEAP库实现的NSGA II算法,要求优化的目标必须大于0,应该怎样设置约束条件
时间: 2024-02-03 11:15:13 浏览: 32
要求优化的目标必须大于0,可以通过设置约束条件来实现,具体步骤如下:
1. 定义一个约束函数,该函数接收一个个体(即一个解)作为参数,并返回一个布尔值,表示该个体是否符合约束条件。
2. 在使用NSGA II算法进行优化时,将约束函数作为参数传递给算法。
3. 当算法生成一个新的个体时,先判断其是否符合约束条件,如果符合,则将该个体添加到候选解集合中;如果不符合,则将该个体舍弃。
例如,假设我们要优化两个目标函数f1和f2,且要求f1和f2都大于0,则可以定义如下的约束函数:
```python
def constraint(individual):
if individual[0] <= 0 or individual[1] <= 0:
return False
else:
return True
```
在使用NSGA II算法时,可以将该约束函数作为参数传递给算法:
```python
from deap import algorithms, base, creator, tools
creator.create("Fitness", base.Fitness, weights=(-1.0, -1.0))
creator.create("Individual", list, fitness=creator.Fitness)
toolbox = base.Toolbox()
toolbox.register("evaluate", evaluate)
toolbox.register("mate", tools.cxSimulatedBinaryBounded, eta=20.0, low=0, up=1)
toolbox.register("mutate", tools.mutPolynomialBounded, eta=20.0, low=0, up=1, indpb=0.2)
toolbox.register("select", tools.selNSGA2)
toolbox.register("constraint", constraint)
toolbox.decorate("mate", tools.DeltaPenalty(toolbox.constraint, 100.0))
toolbox.decorate("mutate", tools.DeltaPenalty(toolbox.constraint, 100.0))
pop = toolbox.population(n=100)
algorithms.eaMuPlusLambda(pop, toolbox, mu=50, lambda_=50, cxpb=0.9, mutpb=0.1, ngen=100)
```
在上述代码中,使用了`tools.DeltaPenalty()`函数将约束函数作为参数传递给了`mate()`和`mutate()`函数,并设置了一个惩罚项的系数,以确保生成的个体都符合约束条件。
相关推荐
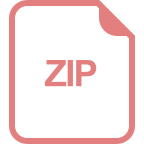
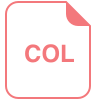













