在本地建立test.txt,依次输出倒数第2、3、4个字符。
时间: 2023-06-11 07:08:02 浏览: 81
你可以使用 Python 编写以下代码:
```
with open("test.txt", "r") as file:
content = file.read()
print(content[-2])
print(content[-3])
print(content[-4])
```
首先使用 `with open("test.txt", "r") as file` 语句打开文件并创建一个文件对象 file,然后使用 `file.read()` 方法读取文件内容并将其存储在变量 content 中。
接下来,我们使用 Python 切片(slice)的方式打印出倒数第 2、3、4 个字符,即 `content[-2]`、`content[-3]`、`content[-4]`。
注意,在读取文件内容时,如果文件中的字符数小于 4,代码会抛出 `IndexError` 异常。因此,在实际应用中,我们需要对这种情况进行处理。
相关问题
定义一个TestFile类要求如下: 1.利用java.nio.file.Files类在E:\myfile路径下创建文件test.txt 2.向该文件写入字符串“我喜欢Java” 3.从test.txt文件中读取字符串,并打印输出 4.按步骤1再创建一个文件test2.txt,并向该文件写入字符串“I hate java” 5.删除文件test2.txt
好的,以下是TestFile类的实现:
```java
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class TestFile {
public static void main(String[] args) {
Path path = Paths.get("E:\\myfile\\test.txt");
Path path2 = Paths.get("E:\\myfile\\test2.txt");
String str1 = "我喜欢Java";
String str2 = "I hate java";
// 创建文件test.txt并写入字符串
try {
Files.createFile(path);
Files.write(path, str1.getBytes(StandardCharsets.UTF_8));
System.out.println("写入文件成功!");
} catch (IOException e) {
e.printStackTrace();
}
// 从test.txt文件中读取字符串并打印输出
try {
String content = Files.readString(path);
System.out.println("读取到文件内容为:" + content);
} catch (IOException e) {
e.printStackTrace();
}
// 创建文件test2.txt并写入字符串
try {
Files.createFile(path2);
Files.write(path2, str2.getBytes(StandardCharsets.UTF_8));
System.out.println("写入文件成功!");
} catch (IOException e) {
e.printStackTrace();
}
// 删除文件test2.txt
try {
Files.deleteIfExists(path2);
System.out.println("删除文件成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这段代码中,我们定义了一个TestFile类,按照题目要求实现了以下功能:
1. 利用Files.createFile()方法创建文件test.txt,并使用Files.write()方法向文件中写入字符串。
2. 使用Files.readString()方法从test.txt文件中读取字符串,并打印输出。
3. 按照步骤1的方式创建文件test2.txt,并向文件中写入字符串。
4. 使用Files.deleteIfExists()方法删除文件test2.txt。
需要注意的是,在写入和读取文件时,我们使用了StandardCharsets.UTF_8编码格式来保证字符集的正确性。
填写程序,有两个文本文件test.1.txt和test.2.txt,各存放一行字符串,要求把这两个文件中的信息合并,按字符顺序排列,输出到一个新文件test.3.txt中,最后关闭文件
以下是Python代码实现:
```python
with open('test.1.txt', 'r') as file1, open('test.2.txt', 'r') as file2, open('test.3.txt', 'w') as file3:
# 读取文件1和文件2中的字符串并合并
merged_str = file1.readline().strip() + file2.readline().strip()
# 按字符顺序排序
sorted_str = ''.join(sorted(merged_str))
# 将排序后的字符串写入文件3中
file3.write(sorted_str)
# 关闭文件
file1.close()
file2.close()
file3.close()
```
这段代码使用了 `with` 语句来打开文件,并自动关闭文件,避免了手动关闭文件的问题。最后按照题目要求将排序后的字符串写入 `test.3.txt` 文件中,并关闭所有文件。
阅读全文
相关推荐












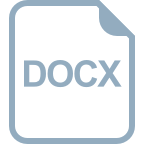