给出解决Toxic Comment Classification Challenge的代码
时间: 2023-03-22 13:00:45 浏览: 62
由于Toxic Comment Classification Challenge是一个Kaggle比赛,我无法提供完整的解决方案代码,但我可以给您提供一些代码片段和建议:
1. 数据探索和预处理:
```
import pandas as pd
# 读取数据
train_df = pd.read_csv("train.csv")
# 查看数据前几行
train_df.head()
# 统计每个标签的数量
label_counts = train_df.iloc[:,2:].sum()
print(label_counts)
# 预处理评论文本数据
import re
import nltk
from nltk.corpus import stopwords
stop_words = stopwords.words("english")
def preprocess_text(text):
# 移除标点符号
text = re.sub(r'[^\w\s]','',text)
# 转换为小写
text = text.lower()
# 移除停用词
text = " ".join([word for word in text.split() if word not in stop_words])
return text
train_df["clean_comment_text"] = train_df["comment_text"].apply(preprocess_text)
```
2. 特征提取和模型训练:
```
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.linear_model import LogisticRegression
from sklearn.multiclass import OneVsRestClassifier
from sklearn.metrics import roc_auc_score
from sklearn.model_selection import train_test_split
# 定义TF-IDF向量化器
vectorizer = TfidfVectorizer(max_features=10000)
# 特征提取和标签
X = vectorizer.fit_transform(train_df["clean_comment_text"])
y = train_df.iloc[:,2:]
# 划分训练集和验证集
X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.2, random_state=42)
# 训练一个多标签逻辑回归模型
clf = OneVsRestClassifier(LogisticRegression(random_state=42))
clf.fit(X_train, y_train)
# 在验证集上进行预测并计算AUC
y_pred_proba = clf.predict_proba(X_val)
auc = roc_auc_score(y_val, y_pred_proba)
print("AUC score: ", auc)
```
3. 对测试数据进行预测:
```
test_df = pd.read_csv("test.csv")
# 预处理测试数据
test_df["clean_comment_text"] = test_df["comment_text"].apply(preprocess_text)
# 提取测试数据的特征
X_test = vectorizer.transform(test_df["clean_comment_text"])
# 进行预测
y_pred_proba_test = clf.predict_proba(X_test)
# 将预测结果保存到CSV文件中
submission_df = pd.DataFrame(data=y_pred_proba_test, columns=y.columns)
submission_df.insert(0, "id", test_df["id"])
submission_df.to_csv("submission.csv", index=False)
```
请注意,这只是一个示例代码,您可以根据自己的数据和模型选择进行调整。
相关推荐
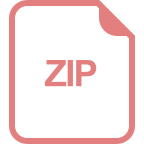
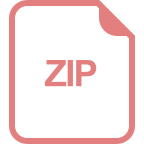













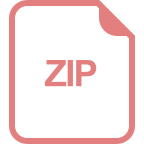