java实现super和this调用
时间: 2024-03-17 10:43:29 浏览: 65
在 Java 中,super 和 this 是关键字,用于调用父类和当前类的成员变量和成员方法。
使用 super 调用父类的成员变量和成员方法,格式为:super.成员变量名或方法名。
例如:
```java
class Animal {
int age = 1;
void eat() {
System.out.println("Animal is eating");
}
}
class Dog extends Animal {
int age = 2;
void eat() {
super.eat(); // 调用父类的 eat 方法
System.out.println("Dog is eating");
}
void printAge() {
System.out.println(super.age); // 调用父类的 age 成员变量
System.out.println(this.age); // 调用子类的 age 成员变量
}
}
public class Test {
public static void main(String[] args) {
Dog dog = new Dog();
dog.eat();
dog.printAge();
}
}
```
使用 this 调用当前类的成员变量和成员方法,格式为:this.成员变量名或方法名。
例如:
```java
class Person {
String name;
int age;
Person(String name, int age) {
this.name = name; // 使用 this 调用当前类的成员变量
this.age = age;
}
void printInfo() {
System.out.println("Name: " + this.name); // 使用 this 调用当前类的成员变量和成员方法
System.out.println("Age: " + this.getAge());
}
int getAge() {
return this.age;
}
}
public class Test {
public static void main(String[] args) {
Person person = new Person("Tom", 20);
person.printInfo();
}
}
```
阅读全文
相关推荐





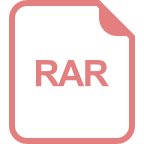








