有一个html表table,要在网页添加个输入框回车后查询在表格第一列是否存在数据,存在整行变绿色背景, 未找到提示出来,并加声音提示,怎么写
时间: 2024-05-05 09:18:51 浏览: 94
您可以使用 JavaScript 编写此功能。首先,使用 DOM 获取表格以及输入框,然后在输入框上添加一个“keydown”事件监听器,监听回车键按下事件。在回调函数中,获取输入框中的文本,遍历表格中的行,查找是否存在匹配的文本。如果找到了,将整行设置为绿色背景;如果没有找到,使用 JavaScript alert() 函数提示用户,并播放提示音。以下是简单的代码示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>Example</title>
<style>
tr.green { background-color: #8BC34A; }
</style>
</head>
<body>
<label>Search:</label>
<input type="text" id="searchBox">
<table id="myTable">
<tr>
<th>Name</th>
<th>Age</th>
<th>Gender</th>
</tr>
<tr>
<td>John</td>
<td>30</td>
<td>Male</td>
</tr>
<tr>
<td>Jane</td>
<td>25</td>
<td>Female</td>
</tr>
<tr>
<td>Bob</td>
<td>40</td>
<td>Male</td>
</tr>
</table>
<audio id="alertSound">
<source src="alert.mp3" type="audio/mpeg">
</audio>
<script>
// Get the table element and search box element
var table = document.getElementById("myTable");
var searchBox = document.getElementById("searchBox");
// Add event listener for search box
searchBox.addEventListener("keydown", function(event){
// Check if enter key was pressed
if(event.keyCode === 13) {
// Get search text
var searchText = event.target.value.toLowerCase();
// Reset table row colors
for(var i=0; i < table.rows.length; i++) {
table.rows[i].classList.remove("green");
}
// Check if search text is empty or whitespace
if(!searchText.replace(/\s+/g, '')) { return; }
// Loop through table rows
for(var i=1; i < table.rows.length; i++) {
var rowText = table.rows[i].cells[0].textContent.toLowerCase();
// If search text is found, set row to green and exit loop
if(rowText.indexOf(searchText) !== -1) {
table.rows[i].classList.add("green");
return;
}
}
// Search text not found, show alert and play sound
alert("Search text not found!");
document.getElementById("alertSound").play();
}
});
</script>
</body>
</html>
```
您可将代码复制到一个 HTML 文件中并在浏览器中打开,则可以在页面上尝试使用该功能。注意:代码示例仅实现了最基本的功能,您可以根据需要进行更改和扩展。
阅读全文
相关推荐
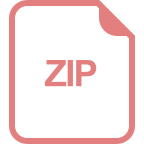
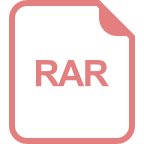


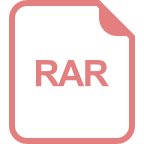
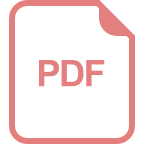
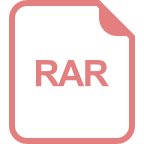
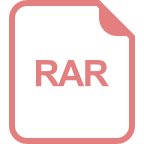









