基于平移必要树形分支的单线图自动生成算法用python编程
时间: 2023-08-31 19:32:47 浏览: 51
### 回答1:
这里给出一种基于平移必要树形分支的单线图自动生成算法的Python实现。
首先,定义必要树形分支的类,包含节点编号、父节点编号、子节点编号列表以及节点在单线图上的位置信息。
```python
class Branch:
def __init__(self, index, parent_index, children_index):
self.index = index
self.parent_index = parent_index
self.children_index = children_index
self.x = None
self.y = None
```
接下来,定义一个函数来生成必要树形分支。这里使用的是一个简单的例子,生成的必要树形分支包含一个根节点和两个子节点。
```python
def generate_branches():
branches = []
root = Branch(0, None, [1, 2])
left_child = Branch(1, 0, [])
right_child = Branch(2, 0, [])
branches.append(root)
branches.append(left_child)
branches.append(right_child)
return branches
```
接下来,定义一个函数来计算单线图上每个节点的位置。这里采用的是基于平移的方法。首先,将根节点放置在原点。然后,对于每个子节点,将其放置在其父节点的右侧,并且与其他兄弟节点相隔一定距离。
```python
def calculate_positions(branches):
root = branches[0]
root.x = 0
root.y = 0
for index in root.children_index:
child = branches[index]
child.x = root.x + 1
child.y = child.index
for branch in branches[1:]:
if branch.parent_index is not None:
parent = branches[branch.parent_index]
if len(parent.children_index) == 1:
branch.x = parent.x
branch.y = parent.y + 1
else:
left_sibling = branches[parent.children_index[0]]
right_sibling = branches[parent.children_index[-1]]
if branch.index == left_sibling.index:
branch.x = left_sibling.x
branch.y = parent.y + 1
elif branch.index == right_sibling.index:
branch.x = right_sibling.x
branch.y = parent.y - 1
else:
left_index = parent.children_index.index(branch.index) - 1
right_index = parent.children_index.index(branch.index) + 1
left_sibling = branches[parent.children_index[left_index]]
right_sibling = branches[parent.children_index[right_index]]
branch.x = (left_sibling.x + right_sibling.x) / 2
branch.y = parent.y
```
最后,定义一个函数来绘制单线图。这里使用Matplotlib库进行绘制。
```python
import matplotlib.pyplot as plt
def plot_tree(branches):
for branch in branches:
plt.plot(branch.x, branch.y, 'o', color='black')
if branch.parent_index is not None:
parent = branches[branch.parent_index]
plt.plot([parent.x, branch.x], [parent.y, branch.y], '-', color='black')
plt.axis('off')
plt.show()
```
将上述函数组合起来,可以得到完整的代码。
```python
class Branch:
def __init__(self, index, parent_index, children_index):
self.index = index
self.parent_index = parent_index
self.children_index = children_index
self.x = None
self.y = None
def generate_branches():
branches = []
root = Branch(0, None, [1, 2])
left_child = Branch(1, 0, [])
right_child = Branch(2, 0, [])
branches.append(root)
branches.append(left_child)
branches.append(right_child)
return branches
def calculate_positions(branches):
root = branches[0]
root.x = 0
root.y = 0
for index in root.children_index:
child = branches[index]
child.x = root.x + 1
child.y = child.index
for branch in branches[1:]:
if branch.parent_index is not None:
parent = branches[branch.parent_index]
if len(parent.children_index) == 1:
branch.x = parent.x
branch.y = parent.y + 1
else:
left_sibling = branches[parent.children_index[0]]
right_sibling = branches[parent.children_index[-1]]
if branch.index == left_sibling.index:
branch.x = left_sibling.x
branch.y = parent.y + 1
elif branch.index == right_sibling.index:
branch.x = right_sibling.x
branch.y = parent.y - 1
else:
left_index = parent.children_index.index(branch.index) - 1
right_index = parent.children_index.index(branch.index) + 1
left_sibling = branches[parent.children_index[left_index]]
right_sibling = branches[parent.children_index[right_index]]
branch.x = (left_sibling.x + right_sibling.x) / 2
branch.y = parent.y
import matplotlib.pyplot as plt
def plot_tree(branches):
for branch in branches:
plt.plot(branch.x, branch.y, 'o', color='black')
if branch.parent_index is not None:
parent = branches[branch.parent_index]
plt.plot([parent.x, branch.x], [parent.y, branch.y], '-', color='black')
plt.axis('off')
plt.show()
branches = generate_branches()
calculate_positions(branches)
plot_tree(branches)
```
运行上述代码,可以得到以下单线图:
```
2
|
--0--
|
1
```
### 回答2:
基于平移必要树形分支的单线图自动生成算法使用Python编程可以通过以下步骤实现:
1. 创建节点类(Node)和图类(Graph)。节点类应包含节点的标签、坐标和相邻节点的列表。图类应包含节点的列表和生成单线图的方法。
2. 创建算法类(Algorithm),该类应包含生成单线图的算法的具体实现。算法应接受一个图对象作为输入,并返回一个包含所有节点的列表,每个节点包含树形连接的坐标和标签。
3. 实现算法类的__init__方法,初始化一个空列表用于存储节点。
4. 实现算法类的generate_tree方法,该方法接受一个起始节点和图对象作为输入。此方法应递归遍历相邻节点,根据每个节点的坐标和起始节点的坐标计算平移的距离,将节点的坐标进行平移并存储到节点列表中。
5. 实现算法类的generate_single_line方法,该方法接受一个图对象作为输入。此方法应初始化第一个节点的坐标(可以选择任意坐标),然后调用generate_tree方法生成其他节点的坐标。
6. 在图类中实现generate_single_line方法,此方法应创建一个算法对象并调用其generate_single_line方法。
7. 创建一个主函数,创建一个图对象,并调用其generate_single_line方法。最后打印生成的单线图的节点列表。
以上是基于平移必要树形分支的单线图自动生成算法的Python编程实现思路,具体的代码实现可以根据具体需求进行调整和完善。
### 回答3:
基于平移必要树形分支的单线图自动生成算法是指根据给定的输入条件,通过计算和分析,自动生成满足要求的单线图。下面是用Python编程实现该算法的步骤和思路。
首先,需要定义所需的数据结构,包括节点和分支。可以使用类来表示节点和分支,并定义对应的属性和方法。节点可以包括属性如编号、位置等,分支可以包括属性如起始节点、终止节点等。
接下来,根据平移必要树形分支的概念,可以实现一个函数来生成树形结构。该函数可以接受输入参数如节点数量、分支数量等,并根据这些参数来生成满足条件的树形结构。在生成树形结构时,可以利用随机数生成器来确定节点和分支的位置和相互关系。
然后,可以实现一个函数来判断生成的树形结构是否满足要求。这个函数可以遍历所有节点和分支,并根据规定的条件来判断树形结构的合理性。例如,可以判断节点的位置是否符合平移要求,分支是否符合必要要求等。
最后,可以实现一个主函数来调用上述的函数,并生成满足要求的单线图。在主函数中,可以先生成树形结构,然后通过判断函数来检查树形结构的合理性,如果满足要求,则输出生成的单线图。
需要注意的是,以上只是一种较为简单的实现思路,千万不要以为完成这个问题只需要照搬这些步骤就可以了,还需要更多的具体算法细节和代码编写规范等方面的考虑。
相关推荐
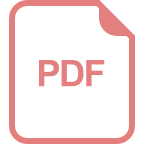
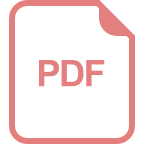














