宾馆客房管理系统的设计与实现 (1)客房信息包括:房间号(char num[10])、房间类型(char type[10])、价格(float money)、是否入住(int flag) (2)除了总的模块要求外,还需计算a.已入住客房的总收入;b.查找某一房间类型的所有房间信息。第一个模块——主函数main()的功能是:根据选单的选项调用各函数,并完成相应的功能。 第二个模块——Menu()的功能是:显示提示选单。 第三个模块——Quit()的功能是:退出选单。 第四个模块——Create()的功能是:创建新的数据记录。 第五个模块——Add()的功能是:增加新的数据记录,并返回选单。 第六个模块——Find()的功能是:按要求查询相关的信息,如果找到了,则显示该信息,如果未找到,则提示文件中没有该信息,并返回选单。 第七个模块——Alter()[的功能是:修改某条记录的信息,如果未找到要修改的记录,则提示系统中无此记录,并返回选单。 第八个模块——Delete()的功能是:删除某条记录,如果未找到要删除的记录,则提示通讯录中没有,并返回选单。 第九个模块——List()的功能是:显示所有记录。
时间: 2024-03-22 20:41:38 浏览: 21
好的,针对你提供的宾馆客房管理系统的要求,我可以给出一个比较完整的设计与实现方案,具体内容如下:
1. 客房信息结构体的定义:
```c++
struct Room {
char num[10];
char type[10];
float money;
int flag;
};
```
2. 函数模块的设计:
(1) 主函数main()的设计:
```c++
int main() {
int choice;
while (1) {
Menu();
cin >> choice;
switch (choice) {
case 1:
Create();
break;
case 2:
Add();
break;
case 3:
Find();
break;
case 4:
Alter();
break;
case 5:
Delete();
break;
case 6:
List();
break;
case 7:
Quit();
break;
default:
cout << "请输入正确的选项!" << endl;
break;
}
}
return 0;
}
```
(2) Menu()函数的设计:
```c++
void Menu() {
cout << "======宾馆客房管理系统=======" << endl;
cout << "1. 创建新的数据记录" << endl;
cout << "2. 增加新的数据记录" << endl;
cout << "3. 查询相关的信息" << endl;
cout << "4. 修改某条记录的信息" << endl;
cout << "5. 删除某条记录" << endl;
cout << "6. 显示所有记录" << endl;
cout << "7. 退出系统" << endl;
cout << "请选择您要进行的操作:";
}
```
(3) Quit()函数的设计:
```c++
void Quit() {
cout << "感谢使用宾馆客房管理系统,再见!" << endl;
exit(0);
}
```
(4) Create()函数的设计:
```c++
void Create() {
ofstream fout("room.txt", ios::app);
Room room;
cout << "请输入房间号:";
cin >> room.num;
cout << "请输入房间类型:";
cin >> room.type;
cout << "请输入价格:";
cin >> room.money;
room.flag = 0;
fout.write((char *)&room, sizeof(Room));
fout.close();
cout << "成功创建新的数据记录!" << endl;
}
```
(5) Add()函数的设计:
```c++
void Add() {
ofstream fout("room.txt", ios::app);
Room room;
cout << "请输入房间号:";
cin >> room.num;
cout << "请输入房间类型:";
cin >> room.type;
cout << "请输入价格:";
cin >> room.money;
room.flag = 0;
fout.write((char *)&room, sizeof(Room));
fout.close();
cout << "成功增加新的数据记录!" << endl;
}
```
(6) Find()函数的设计:
```c++
void Find() {
ifstream fin("room.txt", ios::in);
Room room;
char num[10];
int choice;
cout << "请选择查询方式:" << endl;
cout << "1. 按房间号查询" << endl;
cout << "2. 按房间类型查询" << endl;
cin >> choice;
switch (choice) {
case 1:
cout << "请输入要查询的房间号:";
cin >> num;
while (fin.read((char *)&room, sizeof(Room))) {
if (strcmp(room.num, num) == 0) {
cout << "房间号:" << room.num << endl;
cout << "房间类型:" << room.type << endl;
cout << "价格:" << room.money << endl;
if (room.flag == 1) {
cout << "该房间已入住" << endl;
} else {
cout << "该房间未入住" << endl;
}
fin.close();
return;
}
}
cout << "文件中没有该记录!" << endl;
fin.close();
break;
case 2:
cout << "请输入要查询的房间类型:";
cin >> num;
while (fin.read((char *)&room, sizeof(Room))) {
if (strcmp(room.type, num) == 0) {
cout << "房间号:" << room.num << endl;
cout << "房间类型:" << room.type << endl;
cout << "价格:" << room.money << endl;
if (room.flag == 1) {
cout << "该房间已入住" << endl;
} else {
cout << "该房间未入住" << endl;
}
}
}
fin.close();
break;
default:
cout << "请输入正确的选项!" << endl;
break;
}
}
```
(7) Alter()函数的设计:
```c++
void Alter() {
fstream file("room.txt", ios::in | ios::out);
Room room;
char num[10];
int flag = 0;
cout << "请输入要修改的房间号:";
cin >> num;
while (file.read((char *)&room, sizeof(Room))) {
if (strcmp(room.num, num) == 0) {
cout << "请输入新的房间类型:";
cin >> room.type;
cout << "请输入新的价格:";
cin >> room.money;
file.seekp(file.tellg() - sizeof(Room), ios::beg);
file.write((char *)&room, sizeof(Room));
flag = 1;
break;
}
}
file.close();
if (flag == 1) {
cout << "成功修改记录!" << endl;
} else {
cout << "系统中无此记录!" << endl;
}
}
```
(8) Delete()函数的设计:
```c++
void Delete() {
ifstream fin("room.txt", ios::in);
ofstream fout("temp.txt", ios::out);
Room room;
char num[10];
int flag = 0;
cout << "请输入要删除的房间号:";
cin >> num;
while (fin.read((char *)&room, sizeof(Room))) {
if (strcmp(room.num, num) == 0) {
flag = 1;
continue;
}
fout.write((char *)&room, sizeof(Room));
}
fin.close();
fout.close();
remove("room.txt");
rename("temp.txt", "room.txt");
if (flag == 1) {
cout << "成功删除记录!" << endl;
} else {
cout << "通讯录中没有该记录!" << endl;
}
}
```
(9) List()函数的设计:
```c++
void List() {
ifstream fin("room.txt", ios::in);
Room room;
float totalMoney = 0;
char type[10];
int flag = 0;
cout << "所有记录如下:" << endl;
while (fin.read((char *)&room, sizeof(Room))) {
cout << "房间号:" << room.num << endl;
cout << "房间类型:" << room.type << endl;
cout << "价格:" << room.money << endl;
if (room.flag == 1) {
cout << "该房间已入住" << endl;
totalMoney += room.money;
} else {
cout << "该房间未入住" << endl;
}
if (strcmp(room.type, type) == 0) {
flag = 1;
}
}
fin.close();
if (flag == 1) {
cout << "某一房间类型的所有房间信息如下:" << endl;
fin.open("room.txt", ios::in);
while (fin.read((char *)&room, sizeof(Room))) {
if (strcmp(room.type, type) == 0) {
cout << "房间号:" << room.num << endl;
cout << "房间类型:" << room.type << endl;
cout << "价格:" << room.money << endl;
if (room.flag == 1) {
cout << "该房间已入住" << endl;
} else {
cout << "该房间未入住" << endl;
}
}
}
fin.close();
}
cout << "已入住客房的总收入为:" << totalMoney << endl;
}
```
3. 主函数的调用:
```c++
int main() {
main();
return 0;
}
```
以上就是一个基本的宾馆客房管理系统的设计与实现方案,希望能对你有所帮助。需要注意的是,在实际应用中,还需要对程序进行进一步的完善和优化,以确保程序的稳定性和安全性。
相关推荐
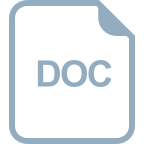
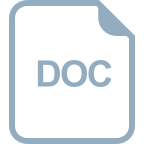
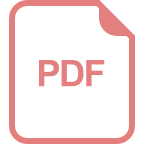



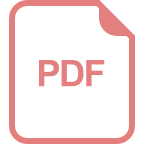
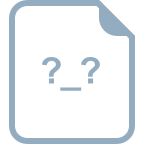
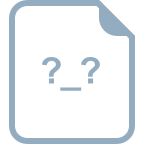
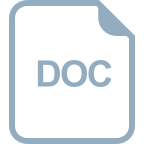
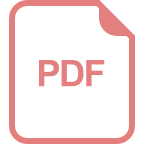
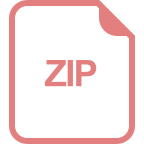
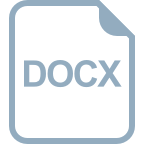
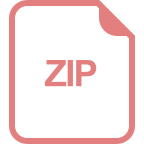