canvas吃豆人小游戏代码
时间: 2023-07-09 22:15:58 浏览: 168
下面是一个简单的canvas吃豆人小游戏的代码示例,仅供参考:
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Canvas吃豆人小游戏</title>
</head>
<body>
<canvas id="canvas" width="400" height="400" style="background-color: black;"></canvas>
</body>
</html>
```
JavaScript代码:
```javascript
// 获取canvas元素
var canvas = document.getElementById('canvas');
var ctx = canvas.getContext('2d');
// 定义小球和豆子的位置
var ball = {x: 200, y: 200, radius: 10, color: 'yellow'};
var dots = [{x: 50, y: 50, radius: 5, color: 'white'},
{x: 100, y: 100, radius: 5, color: 'white'},
{x: 150, y: 150, radius: 5, color: 'white'},
{x: 200, y: 200, radius: 5, color: 'white'},
{x: 250, y: 250, radius: 5, color: 'white'},
{x: 300, y: 300, radius: 5, color: 'white'}];
// 定义吃豆人的初始方向和速度
var direction = 'right';
var speed = 5;
// 定义得分
var score = 0;
// 绘制小球和豆子
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.fillStyle = ball.color;
ctx.beginPath();
ctx.arc(ball.x, ball.y, ball.radius, 0, Math.PI * 2);
ctx.fill();
for (var i = 0; i < dots.length; i++) {
ctx.fillStyle = dots[i].color;
ctx.beginPath();
ctx.arc(dots[i].x, dots[i].y, dots[i].radius, 0, Math.PI * 2);
ctx.fill();
}
}
// 移动小球
function move() {
if (direction === 'right') {
ball.x += speed;
} else if (direction === 'left') {
ball.x -= speed;
} else if (direction === 'up') {
ball.y -= speed;
} else if (direction === 'down') {
ball.y += speed;
}
}
// 判断小球是否碰到了豆子
function checkCollision() {
for (var i = 0; i < dots.length; i++) {
var distance = Math.sqrt(Math.pow(ball.x - dots[i].x, 2) + Math.pow(ball.y - dots[i].y, 2));
if (distance < ball.radius + dots[i].radius) {
dots.splice(i, 1);
score++;
break;
}
}
}
// 显示得分和游戏结束信息
function showScore() {
ctx.fillStyle = 'white';
ctx.font = '20px Arial';
ctx.fillText('得分:' + score, 10, 30);
if (dots.length === 0) {
ctx.fillText('游戏结束', canvas.width / 2 - 50, canvas.height / 2);
}
}
// 监听用户的键盘输入
document.addEventListener('keydown', function(event) {
if (event.keyCode === 37) {
direction = 'left';
} else if (event.keyCode === 38) {
direction = 'up';
} else if (event.keyCode === 39) {
direction = 'right';
} else if (event.keyCode === 40) {
direction = 'down';
}
});
// 刷新画布,实现动画效果
function animate() {
draw();
move();
checkCollision();
showScore();
if (dots.length > 0) {
requestAnimationFrame(animate);
}
}
// 启动游戏
animate();
```
以上是一个简单的canvas吃豆人小游戏的代码示例,你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
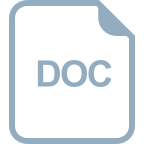
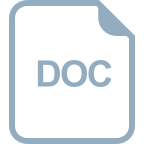
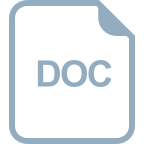
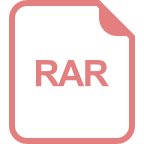
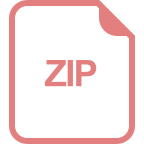
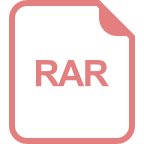
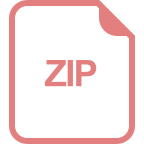
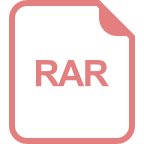
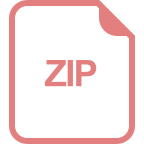
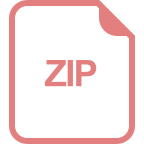
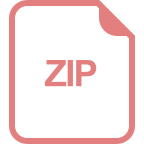
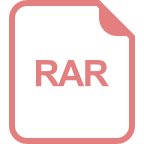
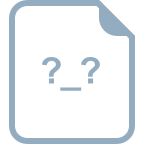
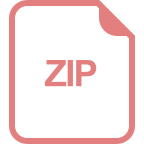
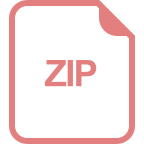
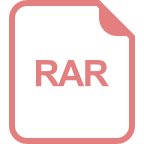
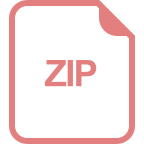
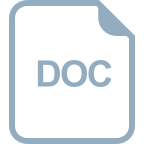
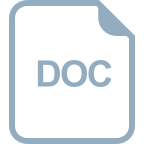