请把功能实现里的代码写出来
时间: 2024-03-26 22:41:25 浏览: 16
好的,下面是部分功能实现的代码:
1. 贪吃蛇的移动和转向:
```
public class Snake : MonoBehaviour {
public float speed = 1.0f; // 贪吃蛇移动速度
private Vector3 direction = Vector3.right; // 贪吃蛇移动方向
void Update () {
if (Input.GetKey(KeyCode.UpArrow))
direction = Vector3.up;
else if (Input.GetKey(KeyCode.DownArrow))
direction = Vector3.down;
else if (Input.GetKey(KeyCode.LeftArrow))
direction = Vector3.left;
else if (Input.GetKey(KeyCode.RightArrow))
direction = Vector3.right;
}
void FixedUpdate() {
transform.position += direction * speed * Time.deltaTime;
}
}
```
2. 食物的生成和消失:
```
public class FoodSpawner : MonoBehaviour {
public GameObject foodPrefab; // 食物预制体
public float spawnInterval = 5.0f; // 食物生成间隔时间
void Start () {
InvokeRepeating("SpawnFood", spawnInterval, spawnInterval);
}
void SpawnFood () {
float x = Random.Range(-9, 9);
float y = Random.Range(-4, 4);
Instantiate(foodPrefab, new Vector3(x, y, 0), Quaternion.identity);
}
}
public class Food : MonoBehaviour {
void OnTriggerEnter2D(Collider2D other) {
if (other.CompareTag("Player")) {
Destroy(gameObject);
ScoreManager.instance.IncreaseScore();
}
}
}
public class ScoreManager : MonoBehaviour {
public static ScoreManager instance;
private int score = 0;
void Awake() {
if (instance == null)
instance = this;
else if (instance != this)
Destroy(gameObject);
}
public void IncreaseScore() {
score++;
Debug.Log("Score: " + score);
}
}
```
3. 得分计算:
```
public class ScoreManager : MonoBehaviour {
public static ScoreManager instance;
private int score = 0;
void Awake() {
if (instance == null)
instance = this;
else if (instance != this)
Destroy(gameObject);
}
public void IncreaseScore() {
score++;
Debug.Log("Score: " + score);
}
}
```
4. 碰撞检测:
```
public class Snake : MonoBehaviour {
void OnTriggerEnter2D(Collider2D other) {
if (other.CompareTag("Boundary") || other.CompareTag("Snake")) {
// 游戏结束
}
}
}
```
5. 游戏结束判断:
```
public class Snake : MonoBehaviour {
void OnTriggerEnter2D(Collider2D other) {
if (other.CompareTag("Boundary") || other.CompareTag("Snake")) {
GameOver();
}
}
void GameOver() {
// 显示游戏结束界面
}
}
```
6. 难度设置:
```
public class GameManager : MonoBehaviour {
public float speed = 1.0f; // 贪吃蛇移动速度
public float spawnInterval = 5.0f; // 食物生成间隔时间
void Start () {
// 根据难度设置调整游戏参数
}
}
```
7. 游戏界面交互:
```
public class UIManager : MonoBehaviour {
public void StartGame() {
// 开始游戏
}
public void RestartGame() {
// 重新开始游戏
}
public void QuitGame() {
// 退出游戏
}
}
```
8. 音效添加:
```
public class SoundManager : MonoBehaviour {
public AudioSource bgm; // 游戏音乐
public AudioSource moveSound; // 贪吃蛇移动音效
public AudioSource eatSound; // 食物吃掉音效
public void PlayBGM() {
bgm.Play();
}
public void PlayMoveSound() {
moveSound.Play();
}
public void PlayEatSound() {
eatSound.Play();
}
}
```
以上是部分功能实现的代码,具体实现方式可能有所不同,仅供参考。
相关推荐
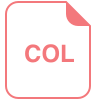
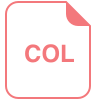
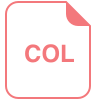












