获取angular 路由配置对象
时间: 2023-09-07 21:04:13 浏览: 131
### 回答1:
你可以使用以下方式来获取 Angular 路由配置对象:
1. 在组件中使用注入的 ActivatedRoute 服务,这个服务会提供当前激活的路由信息。你可以使用 `route.routeConfig` 属性来访问路由配置对象。
```
import { ActivatedRoute } from '@angular/router';
export class MyComponent {
constructor(route: ActivatedRoute) {
console.log(route.routeConfig); // 输出路由配置对象
}
}
```
2. 在模板中使用路由提供的模板变量 `$implicit: Route`,你可以使用 `$implicit.routeConfig` 属性来访问路由配置对象。
```
<ng-template ngFor let-route [ngForOf]="routes">
{{route.routeConfig}}
</ng-template>
```
3. 你也可以使用 Router 服务的 `config` 属性来访问应用中所有的路由配置。
```
import { Router } from '@angular/router';
export class MyComponent {
constructor(router: Router) {
console.log(router.config); // 输出所有的路由配置
}
}
```
### 回答2:
要获取Angular路由配置对象,可以通过注入`Router`服务,并调用其`config`属性来获取。
在Angular中,可以通过在`app.module.ts`中导入`RouterModule`模块来配置路由。在路由配置对象中,使用`Routes`数组来定义各个路由。
假设我们有以下的路由配置:
```typescript
import { Routes, RouterModule } from '@angular/router';
import { HomeComponent } from './components/home/home.component';
import { AboutComponent } from './components/about/about.component';
const routes: Routes = [
{ path: '', component: HomeComponent },
{ path: 'about', component: AboutComponent }
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
```
要获取路由配置对象,可以在任何一个需要它的地方注入`Router`服务,并调用`config`属性来获取路由配置对象。
例如,在一个组件中,可以这样获取路由配置对象:
```typescript
import { Router } from '@angular/router';
import { Component } from '@angular/core';
@Component({
selector: 'app-my-component',
template: `
<ul>
<li *ngFor="let route of routes">{{ route.path }}</li>
</ul>
`
})
export class MyComponent {
routes: any[];
constructor(private router: Router) {
this.routes = this.router.config;
}
}
```
在上面的例子中,我们在组件的构造函数中注入了`Router`服务,并将其赋值给了`router`成员变量。然后我们可以通过调用`this.router.config`来获取路由配置对象,并在模板中使用它。
这样就可以获取到Angular路由配置对象了。
### 回答3:
要获取Angular路由配置对象,需要在Angular的应用中使用Router模块。首先,确保已在应用的根模块中导入了RouterModule,并在imports数组中添加了RouterModule.forRoot(routes)的配置,其中routes是我们定义的路由数组。
在使用路由配置对象前,需要在相关组件中注入Router对象。可以在构造函数中注入,如:
```typescript
constructor(private router: Router) { }
```
然后,通过调用router的config属性,就可以获取到Angular路由配置对象。代码如下:
```typescript
const routeConfig = this.router.config;
```
routeConfig是一个数组,包含了应用中定义的所有路由的配置信息。每个路由配置对象包含了路由路径、路由组件以及其他路由相关的信息。
例如,下面是一个简单的路由配置数组:
```typescript
const routes: Routes = [
{ path: '', redirectTo: '/home', pathMatch: 'full' },
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: 'contact', component: ContactComponent }
];
```
通过this.router.config获取到的路由配置对象数组如下:
```typescript
[
{ path: '', redirectTo: '/home', pathMatch: 'full' },
{ path: 'home', component: HomeComponent },
{ path: 'about', component: AboutComponent },
{ path: 'contact', component: ContactComponent }
]
```
这就是获取Angular路由配置对象的方法。可以根据自己的需要对该配置对象进行操作,例如动态添加或删除路由等。
阅读全文
相关推荐
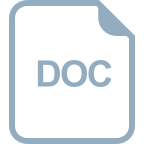
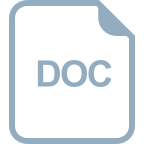
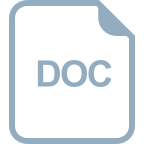
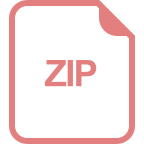
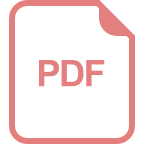
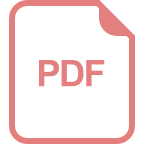
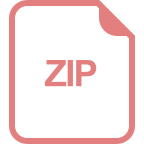
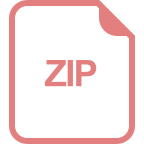
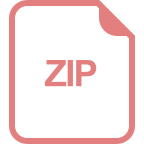
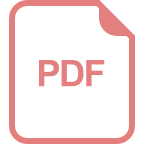
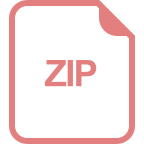
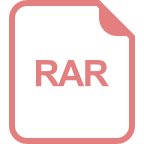
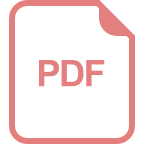
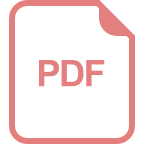
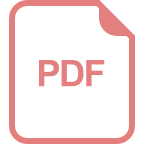
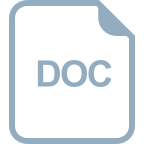
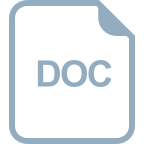
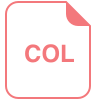
