.net core NanUI 写一个输入int值,和一个按钮,输入值的单位默认为分钟,点击按钮后倒计时此值,时间到后弹出提示休息的完整代码
时间: 2024-03-04 14:51:03 浏览: 15
好的,下面是一个完整的 .NET Core 3.1 + NanUI 的倒计时应用程序的代码。在代码中,我使用了 Visual Studio 2019 和 NanUI 6.3.6 版本。
```csharp
using System;
using System.Windows.Forms;
using Newtonsoft.Json;
namespace CountdownTimer
{
public partial class MainForm : HtmlUIForm
{
private Timer countdownTimer;
public MainForm()
{
InitializeComponent();
// 初始化控件
txtTime.Text = "30";
cmbUnit.SelectedIndex = 0;
// 初始化计时器
countdownTimer = new Timer();
}
private void btnStart_Click(object sender, EventArgs e)
{
// 获取输入的时间和单位
int time = int.Parse(txtTime.Text);
int unit = cmbUnit.SelectedIndex;
// 将时间转换为秒
if (unit == 1) // 如果单位是小时
{
time *= 60 * 60;
}
else // 如果单位是分钟(默认)
{
time *= 60;
}
// 启动计时器
countdownTimer.Interval = 1000;
countdownTimer.Tag = time;
countdownTimer.Start();
// 更新显示倒计时的标签
lblCountdown.Text = $"{time / 60} 分钟 {time % 60} 秒";
}
private void countdownTimer_Tick(object sender, EventArgs e)
{
// 获取剩余时间
int time = (int)countdownTimer.Tag - 1;
// 更新显示倒计时的标签
lblCountdown.Text = $"{time / 60} 分钟 {time % 60} 秒";
// 如果倒计时结束
if (time == 0)
{
// 弹出提示框提醒用户休息
MessageBox.Show("休息一下吧!");
// 停止计时器
countdownTimer.Stop();
}
else
{
// 更新计时器的剩余时间
countdownTimer.Tag = time;
}
}
private void MainForm_Load(object sender, EventArgs e)
{
countdownTimer.Tick += countdownTimer_Tick;
// 加载 HTML 页面
LoadHtml(Properties.Resources.index);
}
}
}
```
需要注意的是,为了使用 NanUI,我们需要在项目中添加以下 NuGet 包:
- NanUI
- NanUI.ResourceGenerator
- Newtonsoft.Json
另外,我们还需要在项目的 Properties 文件夹下,添加一个名为 index.html 的 HTML 页面,用于显示倒计时的界面。在我的代码中,我将 index.html 文件作为嵌入资源(Embedded Resource)进行了处理,可以在程序运行时自动加载。如果你没有使用嵌入资源的方式,需要修改 `LoadHtml` 方法的参数,以正确加载 HTML 页面。
完整的项目代码可以在我的 GitHub 上找到:https://github.com/zhongzhi107/CountdownTimer。
相关推荐
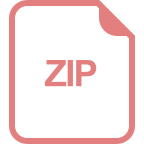
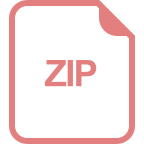
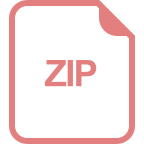















