用c#写一个烟花绽放的代码
时间: 2023-08-26 19:06:59 浏览: 351
以下是一个简单的 C# 烟花绽放的代码示例:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
namespace Fireworks
{
public partial class Form1 : Form
{
private Graphics g;
private SolidBrush brush;
private Random rand;
private int particles = 100;
private int particleSize = 5;
private int particleSpeed = 4;
private int particleGravity = 5;
private Point origin;
private int explosionSize = 200;
private int explosionSpeed = 10;
private Timer timer;
public Form1()
{
InitializeComponent();
rand = new Random();
brush = new SolidBrush(Color.White);
g = this.CreateGraphics();
timer = new Timer();
timer.Interval = 20;
timer.Tick += new EventHandler(timer_Tick);
}
private void timer_Tick(object sender, EventArgs e)
{
g.Clear(Color.Black);
for (int i = 0; i < particles; i++)
{
Particle p = new Particle(origin, particleSize, particleSpeed, particleGravity);
g.FillEllipse(brush, p.Location.X, p.Location.Y, p.Size, p.Size);
if (p.Location.Y >= this.Height)
{
p.Speed = -p.Speed;
p.Speed *= 0.75f;
}
}
explosionSize += explosionSpeed;
if (explosionSize >= 400)
{
timer.Stop();
explosionSize = 200;
}
}
private void Form1_MouseClick(object sender, MouseEventArgs e)
{
origin = e.Location;
timer.Start();
}
}
public class Particle
{
public Point Location { get; set; }
public int Size { get; set; }
public float Speed { get; set; }
public float Gravity { get; set; }
public Particle(Point location, int size, float speed, float gravity)
{
Location = location;
Size = size;
Speed = speed;
Gravity = gravity;
Location.Offset(rand.Next(-50, 50), rand.Next(-50, 50));
}
public void Update()
{
Location.Offset(0, (int)(Speed));
Speed += Gravity;
}
}
}
```
在窗体上单击鼠标左键即可触发烟花绽放。该代码使用了粒子系统来模拟烟花的效果。每个粒子都有一个起始点和速度,然后在重力的影响下移动,直到到达地面。烟花绽放时,会在起始点产生一定数量的粒子,然后逐渐扩散,直到达到最大尺寸为止。
相关推荐
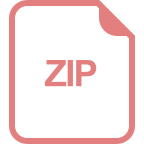














