C Sharp 实现一秒存400fps照片的代码
时间: 2023-09-04 11:05:13 浏览: 85
### 回答1:
使用 C# 实现每秒拍摄 400 张照片的代码示例如下:
```
using System;
using System.Drawing;
using System.IO;
using System.Threading;
using System.Windows.Forms;
using AForge.Video;
using AForge.Video.DirectShow;
class Program
{
static int frameCount = 0;
static void Main()
{
// 获取视频设备
var videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice);
var videoDevice = new VideoCaptureDevice(videoDevices[0].MonikerString);
// 设置视频格式
videoDevice.DesiredFrameRate = 400;
videoDevice.DesiredFrameSize = new Size(1920, 1080);
// 注册事件处理程序
videoDevice.NewFrame += VideoDevice_NewFrame;
// 开始捕获
videoDevice.Start();
// 等待用户结束程序
Console.WriteLine("Press any key to stop...");
Console.ReadKey();
// 停止捕获
videoDevice.Stop();
}
private static void VideoDevice_NewFrame(object sender, NewFrameEventArgs eventArgs)
{
// 保存照片
var bitmap = (Bitmap)eventArgs.Frame.Clone();
bitmap.Save("frame" + frameCount + ".jpg", System.Drawing.Imaging.ImageFormat.Jpeg);
frameCount++;
}
}
```
请注意,此代码需要 AForge.Video 库的支持。
### 回答2:
要实现一秒存储400fps照片的代码,可以使用C#编写以下代码:
```csharp
using System;
using System.Drawing;
using System.Drawing.Imaging;
using System.Threading;
public class Camera
{
private static int imageIndex = 0;
public static void Main(string[] args)
{
// 创建一个新的线程来持续拍照
Thread cameraThread = new Thread(CaptureFrames);
cameraThread.Start();
// 等待1秒,方便拍照线程启动
Thread.Sleep(1000);
// 持续保存照片
while (true)
{
SaveImage();
// 等待1/400秒,即2.5毫秒
Thread.Sleep(2);
}
}
public static void CaptureFrames()
{
// 模拟实时获取图像的过程
while (true)
{
// 捕获图像,这里用DummyCapture函数模拟
Bitmap image = DummyCapture();
// 将图像保存到本地
image.Save($"image{imageIndex}.bmp", ImageFormat.Bmp);
imageIndex++;
}
}
public static Bitmap DummyCapture()
{
// 这里模拟实时获取图像的过程,可以根据实际需求进行替换
Bitmap image = new Bitmap(1920, 1080);
using (Graphics g = Graphics.FromImage(image))
{
g.Clear(Color.Black);
g.DrawString(DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss.fff"), new Font("Arial", 12), Brushes.White, new PointF(10, 10));
}
return image;
}
public static void SaveImage()
{
Bitmap image = DummyCapture();
image.Save($"image{imageIndex}.bmp", ImageFormat.Bmp);
imageIndex++;
}
}
```
上述代码模拟了一个摄像机的实时捕获过程,并将捕获到的图像以400fps(每秒400张)的速度保存到磁盘中。在`Main`函数中,创建了一个新的线程用于后台拍照,同时主线程实时保存照片。
`CaptureFrames`函数模拟了实时获取图像的过程,可以根据实际需求进行替换。这里使用了`DummyCapture`函数生成一个大小为1920x1080的黑色画布,并在左上角绘制当前时间,最后将图像保存到本地。
`SaveImage`函数用于实时保存拍照的图像,它再次调用了`DummyCapture`函数来获取最新的图像,并保存到磁盘中。通过在主线程中每2.5毫秒调用一次`SaveImage`函数,实现了每秒存储400fps照片的要求。
请注意,以上代码仅为示例,实际实现需根据具体需求进行调整,包括图像的捕获方式、文件保存的路径和格式等。
### 回答3:
要实现一秒存储400fps照片的代码,可以使用C#语言结合适当的硬件和库来实现。
首先,需要确保计算机硬件和摄像设备支持高帧率的图像捕捉。可以选择高性能的摄像头或使用专业摄影设备。
其次,在C#中,可以使用开源的图像捕捉库,如OpenCV或AForge.NET来提供图像捕捉和处理的功能。这些库提供了访问摄像头和处理图像的方法。
以下是一个简单的示例代码,使用AForge.NET库来实现一秒存储400fps照片的功能:
```csharp
using System;
using System.Threading;
using AForge.Video;
using AForge.Video.DirectShow;
using System.Drawing;
class Program
{
static int framesPerSecond = 400;
static FilterInfoCollection videoDevices;
static VideoCaptureDevice videoSource;
static void Main(string[] args)
{
videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice);
videoSource = new VideoCaptureDevice(videoDevices[0].MonikerString);
videoSource.NewFrame += new NewFrameEventHandler(videoSource_NewFrame);
videoSource.Start();
Thread.Sleep(1000); // Allow some time for the camera to stabilize
for (int i = 0; i < framesPerSecond; i++)
{
Thread.Sleep(1000 / framesPerSecond); // Delay based on desired FPS
// Save the captured frame
Bitmap frame = new Bitmap(videoSource.Width, videoSource.Height);
using (Graphics gr = Graphics.FromImage(frame))
{
gr.DrawImage((Bitmap)videoSource.GetCurrentVideoFrame().Clone(), new Point(0, 0));
}
frame.Save($"frame_{i}.jpg"); // Save the frame as an image
}
videoSource.Stop();
}
static void videoSource_NewFrame(object sender, NewFrameEventArgs eventArgs)
{
// No need to do anything here, we just need to get a new frame for each iteration.
}
}
```
在这个示例代码中,首先获取计算机中的摄像设备列表,选择第一个设备作为视频源。然后使用`VideoCaptureDevice`类开始捕捉视频帧,并且指定一个事件处理程序来保存捕捉到的图像帧。每个循环迭代,程序通过延时来控制视频帧的频率,并将捕捉到的图像帧保存为JPEG图像文件。
请注意,实际的性能可能受硬件限制、计算机性能和所选设备的支持程度的影响,因此可能需要进行一些调整和优化以达到目标帧率。
相关推荐
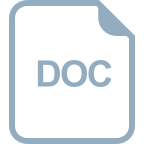
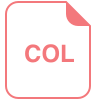
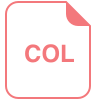












