Java word图片列表导出
时间: 2023-09-24 22:06:10 浏览: 85
您可以使用Apache POI库来实现Java word图片列表导出的功能。下面是一个简单的示例代码,可以将多张图片插入到一个表格中,并将表格导出到Word文档中:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.util.List;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFTable;
import org.apache.poi.xwpf.usermodel.XWPFTableCell;
import org.apache.poi.xwpf.usermodel.XWPFTableRow;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.apache.poi.util.IOUtils;
public class WordImageExport {
public static void exportImageList(List<File> imageList, String outputPath) {
try {
// 创建XWPFDocument对象
XWPFDocument document = new XWPFDocument();
// 创建表格
XWPFTable table = document.createTable(imageList.size(), 1);
// 插入图片
for (int i = 0; i < imageList.size(); i++) {
File imageFile = imageList.get(i);
XWPFTableRow row = table.getRow(i);
XWPFTableCell cell = row.getCell(0);
XWPFParagraph paragraph = cell.getParagraphArray(0);
XWPFRun run = paragraph.createRun();
// 插入图片到段落中
run.addPicture(imageFile, XWPFDocument.PICTURE_TYPE_JPEG, imageFile.getName(), IOUtils.toByteArray(imageFile));
}
// 导出Word文档
FileOutputStream out = new FileOutputStream(outputPath);
document.write(out);
out.close();
System.out.println("Word文档导出成功!");
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
// 图片列表
List<File> imageList = ...; // 从文件夹中读取图片文件
// 导出路径
String outputPath = "output.docx";
exportImageList(imageList, outputPath);
}
}
```
说明:
- 首先,创建XWPFDocument对象表示一个Word文档;
- 然后,使用createTable()方法创建一个表格,表格的行数为图片数量,列数为1;
- 接着,遍历图片列表,使用addPicture()方法将每张图片插入到表格的一个单元格中;
- 最后,将XWPFDocument对象写入到输出流中,导出Word文档。
请注意,上述代码仅为示例代码,您需要根据实际需求进行修改和扩展。
阅读全文
相关推荐
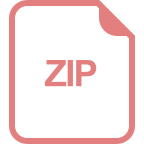
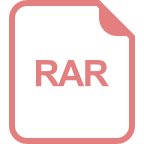
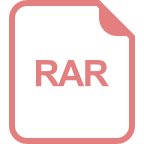
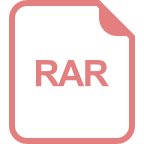
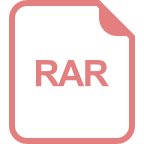
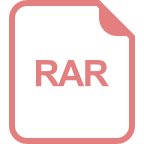
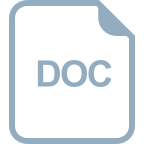

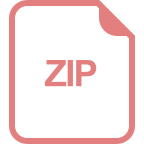
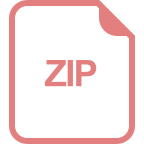
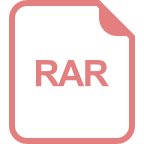
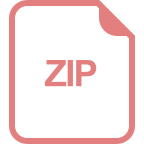
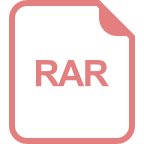
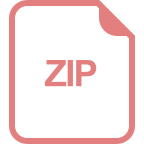
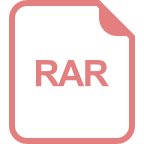
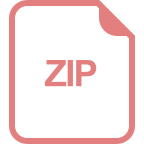