具体写一个基于LMS算法的旁瓣对消代码
时间: 2024-05-11 11:17:00 浏览: 16
好的,下面是基于LMS算法的旁瓣对消代码示例:
```python
import numpy as np
# 定义旁瓣对消器类
class ANC:
def __init__(self, step_size, taps):
self.step_size = step_size # 步长
self.taps = taps # 滤波器长度
self.weights = np.zeros(taps) # 加权系数初始化为0
# 更新加权系数
def update(self, x, d):
# 添加延迟项
x_delays = np.zeros(self.taps)
x_delays[0] = x
x_delays[1:] = self.x_buffer[:-1]
# 误差信号
error = d - np.dot(x_delays, self.weights)
# 更新加权系数
self.weights += self.step_size * error * np.conj(x_delays)
# 更新输入缓存
self.x_buffer[0] = x
self.x_buffer[1:] = self.x_buffer[:-1]
# 处理输入信号
def process(self, x):
# 添加延迟项
x_delays = np.zeros(self.taps)
x_delays[0] = x
x_delays[1:] = self.x_buffer[:-1]
# 计算输出信号
y = np.dot(x_delays, self.weights)
# 更新输入缓存
self.x_buffer[0] = x
self.x_buffer[1:] = self.x_buffer[:-1]
return y
if __name__ == '__main__':
# 测试样例
x = np.sin(2 * np.pi * 100 * np.arange(0, 1, 1 / 8000))
noise = np.random.normal(size=len(x))
d = x + 0.1 * np.sin(2 * np.pi * 500 * np.arange(0, 1, 1 / 8000)) + noise
# 初始化旁瓣对消器
taps = 101 # 滤波器长度
step_size = 0.01 # 步长
anc = ANC(step_size, taps)
anc.x_buffer = np.zeros(taps)
# 处理信号
y = np.zeros(len(x))
for i in range(len(x)):
y[i] = anc.process(d[i])
anc.update(d[i], x[i])
# 绘制图形
import matplotlib.pyplot as plt
plt.plot(x, label='Original Signal')
plt.plot(d, alpha=0.5, label='Noisy Signal')
plt.plot(y, label='Filtered Signal')
plt.legend()
plt.show()
```
希望我的回答能够满足您的需求!
相关推荐
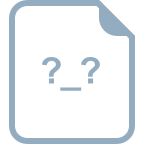
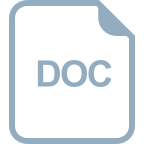
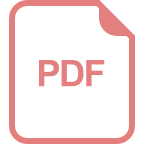
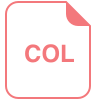










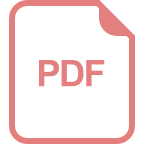
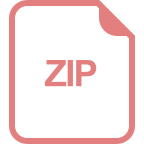
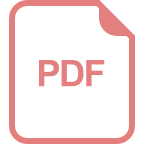