data.loc[:,data.dtypes.values=="int64"].shape[1]
时间: 2024-01-14 19:04:30 浏览: 205
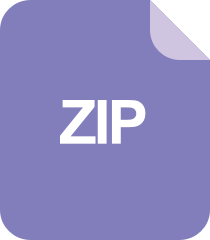
pirosmani.loc:基于视频的网站布局
这段代码是用于获取DataFrame中整型数据列的数量。其中,`data.dtypes`返回了DataFrame中每一列数据的数据类型,`.values`将其转化为一个NumPy数组,`=="int64"`会返回一个布尔型数组,用于过滤出数据类型为int64的列,最后使用`.shape[1]`获取满足条件的列的数量。
可以将这段代码拆分为以下几步:
```python
# 获取DataFrame中每一列数据的数据类型
dtypes = data.dtypes
# 将数据类型转化为NumPy数组
dtypes_array = dtypes.values
# 获取数据类型为int64的列
int64_columns = dtypes_array == "int64"
# 获取满足条件的列的数量
num_int64_columns = int64_columns.sum()
```
或者更简单地:
```python
num_int64_columns = (data.dtypes.values == "int64").sum()
```
注意,这段代码中需要先导入pandas库,因为DataFrame是pandas库中的一种数据结构。
阅读全文
相关推荐
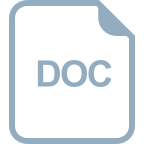
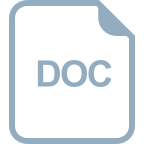




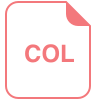
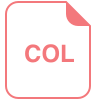







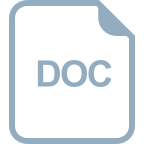
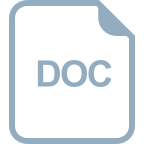