python如何操作mysql
时间: 2023-05-14 22:07:17 浏览: 68
Python可以使用MySQL Connector/Python来操作MySQL数据库。以下是一个简单的示例代码:
```python
import mysql.connector
# 连接数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
# 创建游标对象
mycursor = mydb.cursor()
# 执行SQL查询
mycursor.execute("SELECT * FROM customers")
# 获取查询结果
myresult = mycursor.fetchall()
# 输出查询结果
for x in myresult:
print(x)
```
在这个示例中,我们首先使用`mysql.connector`模块连接到MySQL数据库。然后,我们创建一个游标对象,该对象用于执行SQL查询并获取结果。最后,我们使用`fetchall()`方法获取所有查询结果,并使用循环输出每个结果。
请注意,您需要将`yourusername`和`yourpassword`替换为您的MySQL用户名和密码,并将`mydatabase`替换为您要连接的数据库名称。
相关问题
python 操作mysql
Python可以使用多种库来操作MySQL数据库,其中包括PyMySQL和mysqlclient。PyMySQL是一个纯Python编写的库,安装非常简单,而mysqlclient是底层使用C编写的库,安装可能会有一些问题。\[3\]你可以根据自己的需求选择其中之一进行安装和使用。
在使用PyMySQL库时,你可以使用%s作为参数占位符来执行SQL操作,这与Python自带的sqlite3模块的占位符问号(?)有所不同。如果需要更详细的文档,你可以参考PyMySQL的官方文档。\[2\]
如果你想了解更多关于Python操作MySQL的知识,可以参考一些专门的章节或教程,这些资源会更详细地介绍如何使用Python来进行MySQL数据库操作。\[1\]希望这些信息对你有所帮助!
#### 引用[.reference_title]
- *1* [Python操作之MySQL](https://blog.csdn.net/sanylove/article/details/124166373)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [太全了——用Python操作MySQL的使用教程集锦](https://blog.csdn.net/m0_59485658/article/details/126364328)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [Python操作MySql](https://blog.csdn.net/PAN_BING/article/details/120812542)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python操作MySQL
Python可以使用pymysql库来操作MySQL数据库。首先,需要创建一个连接对象,指定数据库的主机、端口、用户名、密码、数据库名称和字符集等信息。然后,通过该连接对象获取游标对象,可以使用该游标对象执行SQL语句。最后,需要手动提交事务或者回滚,并关闭连接释放资源。
以下是一个示例代码,演示了如何使用pymysql库连接MySQL数据库并插入一条数据:
```python
import pymysql
# 创建连接
conn = pymysql.connect(host='localhost', port=3306, user='root', password='123456', database='hrs', charset='utf8mb4')
try:
# 获取游标对象
with conn.cursor() as cursor:
# 执行SQL语句
affected_rows = cursor.execute('INSERT INTO tb_dep VALUES (%s, %s, %s)', ('1001', '孙悟空', '花果山'))
if affected_rows == 1:
print('添加成功')
# 手动提交事务
conn.commit()
except pymysql.MySQLError:
# 手动回滚事务
conn.rollback()
finally:
# 关闭连接释放资源
conn.close()
```
以上代码首先创建了一个连接对象,然后使用该连接对象获取游标对象。接着,使用游标对象执行了一条插入数据的SQL语句,并判断是否插入成功。最后,手动提交事务并关闭连接。
希望以上信息对您有帮助。如果还有其他问题,请随时提问。
#### 引用[.reference_title]
- *1* *3* [Python操作之MySQL](https://blog.csdn.net/sanylove/article/details/124166373)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down28v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [Python操作MySql](https://blog.csdn.net/PAN_BING/article/details/120812542)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down28v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
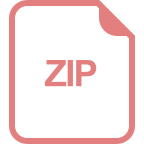












